Welcome to our guide on avoiding common React js mistakes and how to avoid them. As a developer, it’s important to understand the common pitfalls in React js programming to improve your coding skills and produce high-quality code. By avoiding these mistakes, you can create efficient, effective, and scalable React js applications that meet your end-users’ needs and expectations.
Throughout this article, we will cover a range of common React js mistakes and provide expert tips on how to avoid them. Our goal is to help you become a better React js developer by identifying common mistakes and providing you with best practices.
Whether you’re new to React js or a seasoned professional, we hope that this guide will help you improve your React js programming skills and build better applications. So, let’s dive in and explore the most common React js mistakes you should avoid to take your coding skills to the next level.
It is important to remember that avoiding common React js mistakes may take practice, effort, and time, but the end result is well worth it. Keep these tips in mind as you move forward in your coding journey, and you’ll be well on your way to becoming an expert in React js development.
So, let’s get started by exploring the common React.js mistakes and how to avoid them to enhance your React.js development skills.
Keywords: Common React js Mistakes, Avoiding React js Mistakes
Common React js Mistakes and How to Avoid Them
Not Using PropTypes
When building components in React, it’s important to verify that the data passed to them is of the correct type. This is where PropTypes come into play. Unfortunately, many developers overlook this crucial step, leading to tough-to-debug errors in their apps.
The Importance of PropTypes
PropTypes are used to type-check your components and ensure the data passed to them matches the expected type. They offer a means to catch issues before they reach the production stage, making your code more robust and less prone to errors.
Implementing PropTypes in React
Implementing PropTypes is a straightforward process. You can do it using the prop-types package provided by Facebook. First, install the package using the npm package manager. Next, import it into your component and add a propTypes object specifying the type of each property you expect.
Here’s an example:
import PropTypes from 'prop-types';
function MyComponent(props) {
return (
<div>
<h1>{props.title}</h1>
<p>{props.description}</p>
</div>
)
}
MyComponent.propTypes = {
title: PropTypes.string.isRequired,
description: PropTypes.string.isRequired,
}
Improper Component State Management
One of the most critical aspects of React js development is managing the state of your components. Improper management of component state can lead to a host of issues, including bugs, poor app performance, and decreased readability of your code.
One common mistake is failing to keep component state separate from other stateful data in your app. To avoid this mistake, it’s essential to keep your stateful data as close to the component that uses it as possible. This practice helps to ensure that components remain maintainable and scalable over time.
Another common mistake with component state management is relying too heavily on component lifecycle methods like componentDidUpdate. While these methods can be helpful for updating component state, they can also lead to convoluted code and bugs. Instead, consider using hooks like useState and useEffect, which are designed to handle state management in a more streamlined manner.
It’s also vital to keep your component state as simple as possible. Avoid storing unnecessary data in state, which can cause your app to slow down and become more difficult to maintain. Instead, try to pass props down to child components whenever possible, as this reduces the overall complexity of your app and makes it easier to reason about.
Finally, make sure to test your component state thoroughly. This includes both unit testing and integration testing, which can help ensure that your state management code works as intended and doesn’t introduce any new bugs or issues.
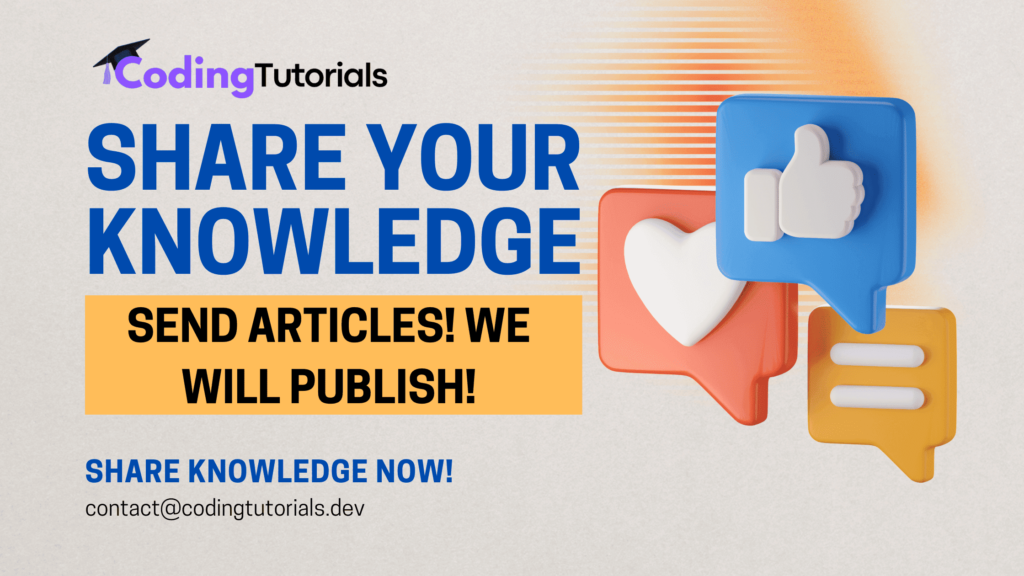
Overusing or Misusing React Components
React components are the building blocks of a React js application, but overusing or misusing them can lead to code duplication and poor performance. To avoid this, it’s important to understand how to create reusable components and abstract them effectively.
Creating Reusable Components
One of the biggest benefits of React components is their reusability. By creating components that can be easily reused throughout your application, you can save time and reduce the risk of errors. When creating reusable components:
- Identify common functionality that can be abstracted into a component
- Ensure components are independent and self-contained
- Use props to pass data and functions between components
Abstracting Components Effectively
While creating reusable components is important, it’s equally important to abstract them effectively. Over-abstracting can lead to confusion and unnecessary complexity. When abstracting components:
- Identify common functionality that can be shared between components
- Avoid creating unnecessary abstractions
- Use component composition rather than inheritance
By creating and abstracting components effectively, you can improve the structure and maintainability of your React js codebase.
Inefficient Rendering
React’s virtual DOM is a powerful feature that can significantly enhance rendering performance. However, it can still be misused, leading to inefficient rendering.
Common Mistakes in React Rendering Optimization
- Rendering too often, even when it’s not necessary
- Using expensive operations in the render method
- Not using shouldComponentUpdate() or React.memo() to prevent unnecessary updates
By avoiding these common mistakes, you can improve your React app’s performance and reduce unnecessary re-renders.
Tips for React Rendering Optimization
- Use shouldComponentUpdate() or React.memo() to prevent unnecessary updates
- Use PureComponent instead of Component for simple components that don’t rely on state or props
- Avoid using arrow functions in the render method, as they create a new reference on every render
By following these tips, you can ensure that your React app renders efficiently and performs well.
Not Handling Errors Properly
Errors can be a significant source of frustration for developers and can lead to buggy React js applications. Proper error handling is essential to ensure that your React app runs smoothly. Unfortunately, many developers make the mistake of not handling errors correctly, which can lead to poor user experiences.
React Error Boundaries
One effective technique for error handling in React is the use of Error Boundaries. Error Boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the crashed component tree. Using Error Boundaries can prevent an entire application from crashing due to a single error in a single component.
Here’s an example of how to use Error Boundaries in your React code:
- Create an ErrorBoundary component that extends the React.Component class and implements the static method getDerivedStateFromError().
- Wrap your components that may throw errors with the ErrorBoundary component.
- Use the componentDidCatch() lifecycle method in your ErrorBoundary component to handle errors and render a fallback UI.
By using Error Boundaries and other error handling techniques, you can prevent crashes and provide a better user experience for your React js application.
Inadequate Component Lifecycle Management
React components have a lifecycle that can be utilized to manage state and perform actions at specific stages of the component’s existence. However, overlooking this lifecycle can result in poor performance and errors.
Class Components and useEffect
Class components have the traditional lifecycle methods like componentWillMount, componentDidMount, componentWillUnmount, and many others. As of React 16.8, functional components can use the useEffect hook to manage component lifecycle.
The useEffect hook can be used to execute functions when a component mounts, updates, and unmounts. It also has the advantage of allowing multiple useEffect hooks in a single component, whereas class components only have one method for each lifecycle event.
It’s important to remember to clean up any resources created during a component’s lifecycle. For instance, if you use an event listener in a component’s componentDidMount, you should remove that listener in the componentWillUnmount method to avoid memory leaks.
Don’t rely on componentWillReceiveProps
componentWillReceiveProps is often misused and can lead to unnecessary re-renders of components. It’s recommended to embrace the concept of “unidirectional data flow” and avoid relying on this method.
In summary, it’s essential to understand the component lifecycle in React js and the tools available to manage it. By utilizing class components or the useEffect hook correctly, you can ensure efficient rendering and improve your application’s performance.
Inconsistent Code Styling: How to Ensure Consistent Coding Conventions
Inconsistent code styling can make React js code difficult to read and maintain. It’s important to follow consistent coding conventions to ensure code readability and maintainability.
Code Styling
Code styling includes consistent indentation, naming conventions, and code structure. The inconsistency in code styling can be reduced by setting rules and guidelines for the team to follow.
ESLint
ESLint is a linter tool used for static code analysis, which can detect code styling inconsistencies and errors. It enforces the coding conventions and provides real-time feedback during development.
Prettier
Prettier is a code formatter that automatically formats the code according to the set rules and guidelines. It ensures consistent code styling and saves time by removing the need for manual formatting.
By using tools like ESLint and Prettier, you can enforce consistent coding conventions and reduce the risk of inconsistent code styling in your React.js projects.
Not Optimizing React Routing
Routing is an essential part of most React js applications. It provides users with a seamless flow between different pages and components. However, inefficient routing can negatively impact the performance of your application.
The Solution: React Router
To optimize routing performance, we recommend using React Router, a powerful routing library for React.js. It allows you to manage your application’s routing declaratively and efficiently. React Router allows for dynamic routing, code splitting, and lazy loading, which can lead to significant performance improvements in your application.
Best Practices for Routing Performance
Here are some additional tips to ensure that your React js routing is optimized for performance:
- Use dynamic imports and code splitting to reduce the initial load time of your application.
- Avoid rendering unnecessary components when navigating between routes.
- Implement pagination to handle large data sets more efficiently and reduce server load.
- Use React Router’s HOC (Higher Order Component) to handle authentication and authorization before rendering specific pages or components.
By following these best practices and using React Router, you can significantly improve the performance of your React js routing. Remember to test your routing thoroughly to ensure that it is functioning correctly and efficiently.
Not Optimizing React Testing and Debugging
React js development involves not only coding but also testing and debugging to ensure a bug-free application. Neglecting proper testing and debugging can lead to various problems. This section will discuss common mistakes in testing and debugging React code and provide strategies for effective testing and debugging.
Not Writing Automated Tests
One of the biggest mistakes developers make is not writing automated tests. Writing automated tests is crucial in producing bug-free React js applications. Automated tests allow developers to detect errors early and ensure that their code is working as expected.
Not Using Debugging Tools
Another common mistake developers make is not using debugging tools. React.js provides excellent debugging tools such as React Developer Tools, which allows developers to inspect and debug their React components. Additionally, using console.log statements can help locate errors and debug React components effectively.
Not Testing for Edge Cases
Testing only the normal use cases is another mistake in React js development. It’s vital to test for edge cases, which are scenarios that may not occur frequently, but when they do, can cause significant problems. Testing edge cases can help ensure that the React.js application is stable and has no errors in even uncommon scenarios.
Not Prioritizing Debugging
Debugging should be given equal importance as coding to produce a high-quality React js application. Many developers do not prioritize debugging and only focus on writing code. Prioritizing debugging can lead to a more efficient development process and a higher quality end-product.
Poor Performance with Large Data Sets
When it comes to React js applications, handling large data sets can be a challenge.
One common mistake is not optimizing React performance for large data sets, resulting in slow rendering times and compromised user experiences.
To improve performance, one approach is pagination. This involves breaking up the data into smaller chunks and rendering only a certain amount at a time.
Using Pagination
Using pagination can significantly improve your React performance. By breaking up data into smaller, more manageable chunks, you reduce the amount of data that needs to be rendered at any given time.
You can implement pagination in several ways, including:
- Using React libraries like React Paginate or React Bootstrap Pagination
- Manually slicing the data and rendering it in parts using state and map() functions
Optimizing with React.memo()
Another way to optimize React performance with large data sets is by using the React.memo() function. This function helps prevent unnecessary re-renders by comparing the current props to the previous props and rendering only when there are changes.
Conclusion
As a React.js developer, avoiding common mistakes is crucial to creating high-quality applications. By understanding the pitfalls we covered in this article and following best practices such as implementing PropTypes, properly managing component state, creating reusable components, and optimizing routing and rendering, you can improve your React js skills and create performant and scalable applications.
Don’t forget the importance of proper error handling, testing, and debugging, as neglecting these areas can lead to buggy applications. Additionally, efficient handling of large data sets is essential to maintain good performance.
Continuously staying up-to-date with the latest React js ecosystem developments and trends can help you excel in your coding journey. Remember, by following these best practices and learning from mistakes, you can create top-notch React.js applications. Happy coding!
Next Tutorial: Exploring Context API in React Js: With easy steps
1 Comment