Welcome to our comprehensive guide on Event Driven Architecture (EDA) in Node.js! In this section, we will introduce you to the world of EDA and explore its benefits and relevance in modern software development.
EDA is a powerful design pattern that enables applications to respond to events and triggers in a fast and efficient way. It has become increasingly popular in recent years due to its scalability and flexibility, making it an essential skill to have as a modern developer.
In this guide, we will cover all aspects of Event Driven Architecture in Node js, from the core principles to real-world use cases. We will explain the event-driven design pattern and how it is implemented in Node.js. You will learn how to structure your code to emit and handle events, as well as how to design scalable microservices using EDA.
If you are looking to enhance your skills in event-driven programming in Node.js, then this guide is for you. By the end of this comprehensive guide, you will have a solid understanding of Event Driven Architecture in Node js, and the skills to build efficient and scalable applications using EDA. Let’s get started!
SEO keywords: event driven architecture in node js, node.js event driven architecture, event-driven architecture, event-driven design pattern, event-driven programming in node js.
Understanding Event Driven Architecture
Welcome to the world of event-based architecture in Node js! In this section, we will provide you with a comprehensive explanation of event-driven architecture (EDA) and its importance in modern software development.
Event-driven system in Node.js is a popular design pattern that involves the use of events to communicate between different components of a system. In this model, events are triggered based on specific actions, and the corresponding handlers are executed accordingly.
What is Event Driven Architecture?
Event Driven Architecture is a software design pattern that emphasizes the use of events to trigger and communicate between different components of a system. It is based on the concept of event-driven programming, which involves the use of events to trigger actions in a program.
In event-driven architecture, components of a system are decoupled and communicate through events. This allows for a more flexible and scalable architecture that can better handle complex systems.
The Core Principles of Event Driven Architecture
Event-driven architecture is based on several core principles that ensure its effectiveness:
- Asynchronous processing: Events are processed asynchronously, ensuring that the system can handle multiple requests simultaneously.
- Decoupling of components: Components of a system are decoupled, which makes it easier to modify and scale the system.
- Loose coupling: Components in an event-driven architecture are loosely coupled, which means that changes to one component will not affect the others.
- Scalability: Event-driven architecture is highly scalable, making it ideal for handling large and complex systems.
The Components of an Event Driven System
The core components of an event-driven system are:
- Event: An event is a trigger that initiates an action in the system.
- Event emitter: An event emitter is responsible for emitting events when specific actions occur.
- Event handler: An event handler is responsible for handling events and executing the corresponding actions.
Real-World Examples of Event Driven Architecture
Event-driven architecture is widely used in modern software development, particularly in microservices architecture. Some popular examples of event-driven systems include:
Company | Product/Service |
---|---|
Netflix | Content Delivery Network (CDN) |
Uber | Real-time location-based system |
Airbnb | Booking and reservation system |
Best Practices for Event Driven Architecture
When implementing event-driven architecture, it’s important to follow best practices to ensure a scalable and maintainable system:
- Use descriptive event names: Use names that clearly identify the event and its purpose.
- Version your events: Use version numbers to help manage changes to events over time.
- Handle errors: Implement error handling mechanisms to ensure proper handling of errors.
- Write tests: Use automated tests to ensure that your event-driven system is functioning properly.
Now that you have a better understanding of event-driven architecture, let’s move on to the key concepts in Node.js event-driven architecture.
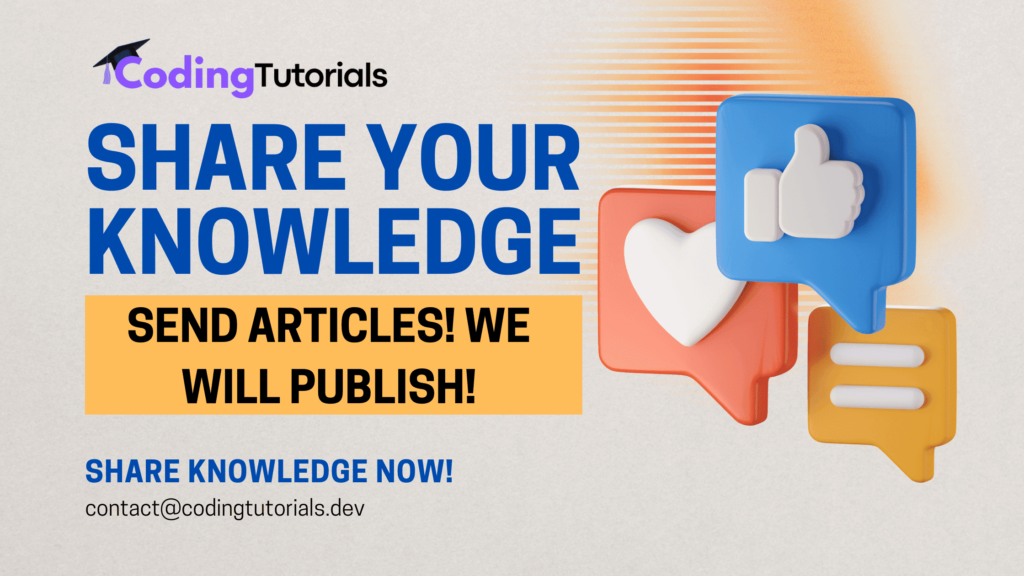
Key Concepts in Node.js Event Driven Architecture
Event Driven Architecture (EDA) revolves around events and how they are handled by various components of a system. In Node.js, events are an essential part of EDA that allows developers to build scalable and efficient applications. To fully harness the power of EDA, it’s essential to understand two key concepts in Node.js: event listeners and event handling.
Node.js Event Listeners
Event listeners are functions that listen for specific events to occur in a Node.js application. They are responsible for handling events emitted by various components of the application. When a listener detects an event, it executes a predefined action. The on() method is used to register an event listener in Node.js.
Let’s consider a real-world example that demonstrates the use of event listeners in Node.js. Imagine a scenario where a user submits a form on a web page. When the user submits the form, an event is triggered, and a listener registered with the form element detects the event. The listener then executes an action, such as sending the form data to a server for processing.
Node.js Event Handling
Event handling refers to the process of handling events in a Node.js application. It involves registering listeners for events and defining how those events are handled. Event handling is a critical component of EDA as it determines how events are processed in a system.
Node.js provides the EventEmitter class to handle events. The class is used to implement event handling in Node.js applications. To handle events using the EventEmitter class, developers can use the emit() method to emit events and the on() method to register listeners for events.
Example
Event | Action |
---|---|
button-click | Shows alert message |
form-submit | Sends form data to a server for processing |
In the example above, we have two events – button-click and form-submit. When a user clicks a button on a web page, the button-click event is emitted. A listener registered for the event detects it and executes an action, such as showing an alert message. Similarly, when a user submits a form, the form-submit event is emitted, and a listener registered for the event sends the form data to a server for processing.
By understanding event listeners and event handling in Node.js, developers can build efficient and scalable applications using EDA.
Building Event Driven Microservices in Node.js
Microservices architecture has gained popularity for its ability to simplify the development and maintenance of complex applications. However, scaling microservices to meet demand can pose a significant challenge. This is where Event Driven Architecture (EDA) can help.
By using event-driven communication between microservices, you can build a scalable and modular system that can quickly adapt to changing loads. In this section, we will explore how to build event-driven microservices in Node.js.
Benefits of Event Driven Microservices
Event-driven microservices architecture offers several benefits, including:
- Scalability – By decoupling the services, you can scale each service independently, ensuring that the system can handle increasing loads.
- Reliability – Event-driven microservices can quickly recover from failures, ensuring the system remains available and responsive.
- Modularity – Each microservice can be developed, deployed, and maintained independently, promoting a more agile development process.
- Flexibility – By using events as a communication mechanism, you can easily introduce new functionality or services without impacting the entire system.
Designing Event Driven Microservices
When designing event-driven microservices, it’s essential to focus on the following:
- Identify events – Determine the events that need to be emitted and subscribed to by each service.
- Define contracts – Define the contracts between services, including the information that will be passed in each event.
- Handle exceptions – Plan for how exceptions will be handled and how the system will recover from errors.
- Ensure consistency – Ensure that the data remains consistent between services by using event sourcing, snapshots, or other techniques.
Let’s take a look at an example of how to implement event-driven microservices architecture in Node.js.
Example: Building Event Driven Microservices with Node.js
In this example, we will build two microservices: “Inventory” and “Order”. The Inventory microservice will manage the inventory, and the Order microservice will manage customer orders. When a customer places an order, the Order service will emit an “OrderPlaced” event, which the Inventory service will subscribe to, reducing the available inventory count.
Event | Data |
---|---|
OrderPlaced | Order ID, Product ID, Quantity |
InventoryUpdated | Product ID, Quantity |
As shown in the table above, the “OrderPlaced” event contains the necessary data for the Inventory service to reduce the available inventory count. Once the Inventory service updates the inventory count, it will emit an “InventoryUpdated” event, which can be used by other services that rely on inventory data.
To implement our services, we can use a library like Seneca.js, which simplifies the development of microservices.
Here’s an example of the Inventory microservice:
const seneca = require('seneca')();
// Subscribe to OrderPlaced event
seneca.add({ role: 'inventory', cmd: 'reduceStock' }, (msg, respond) => {
const { productId, quantity } = msg;
// Update inventory
// ...
// Emit InventoryUpdated event
seneca.act({ role: 'inventory', cmd: 'updateInventory', productId, quantity }, respond);
});
// Start the service
seneca.listen({ port: 9001, pin: { role: 'inventory' } }, () => {
console.log('Inventory Service Running on port 9001');
});
And here’s an example of the Order microservice:
const seneca = require('seneca')();
// Emit OrderPlaced event
seneca.act({ role: 'inventory', cmd: 'reduceStock', productId, quantity }, (err, response) => {
if (err) {
// Handle error
return;
}
// Handle response
});
// Start the service
seneca.listen({ port: 9002, pin: { role: 'order' } }, () => {
console.log('Order Service Running on port 9002');
});
As shown in the examples, each microservice can emit and subscribe to events, allowing for a decoupled and scalable system.
Event Sourcing in Node.js
When building complex systems, maintaining data consistency becomes a critical challenge. Traditional approaches rely on databases to store and retrieve the current state of the system. However, this approach can limit scalability and processing speed.
Event Sourcing provides an alternative solution by storing a log of all actions or events that occur in the system. This approach defines the system’s state at any point by replaying these events, allowing for a complete audit trail of changes and the ability to roll back to previous states.
In Node.js, we can implement event sourcing by defining events as objects that encapsulate data related to state changes and persist them to a database or log. These events can be replayed to reconstruct the current state of the system.
Benefits of Event Sourcing in Node.js
Event Sourcing provides several benefits, including:
- Improved data consistency: By recording every state-changing event, we can recreate the current state, ensuring data consistency and accuracy.
- Easier debugging: With a complete audit trail of changes, identifying and resolving issues is more straightforward.
- Enhanced scalability: Since event sourcing only stores immutable events, it can handle large datasets and easily accommodate new data sources without affecting existing data.
Implementing Event Sourcing in Node.js
To implement event sourcing in Node.js, we need to define the events, persist them, and reconstruct the system’s state. The following table illustrates the steps involved:
Step | Description |
---|---|
Define Events | Create objects that represent events and encapsulate data related to state changes. |
Persist Events | Store events in a database or log. |
Recreate State | Read stored events and use them to recreate the system’s current state. |
Implementing this process requires careful consideration of event storage and retrieval mechanisms, serialization, and replay strategies. However, Node.js provides several libraries and tools that simplify event sourcing implementation, such as EventStore and Eventide.
By leveraging Event Sourcing in your Node.js applications, you can ensure data consistency, facilitate debugging, and enhance scalability, ensuring your system can handle large datasets and new data sources without affecting existing data.
Implementing Event Driven Architecture in Node js
Implementing Event Driven Architecture in Node js requires a few essential steps. Below we have outlined the necessary actions required to structure your application for event-driven workflows.
Step 1: Define Events
The first step is to define the events that your application will emit. Properly naming the events is essential for the effective handling of events. Also, consider the various states that an event can trigger; it will help you to identify the correct event name for your application.
Step 2: Create Event Handlers
Next, we create event handlers; these are the functions that execute when an event is emitted. Node.js provides the eventEmitter.on() function to create event handlers for specific events. Develop unique handlers for each event that is defined in step 1.
Step 3: Emit Events
To emit events from your application, you can use the eventEmitter.emit() method. Emit the event name that represents the change or action in your application.
Step 4: Handle Errors
Handling errors is crucial in event-driven programming. Use try-catch blocks to handle errors, avoiding the application from breaking. The event emitter also provides an error event to help catch and handle errors.
Here’s an example event-emitter code snippet:
//Import the event module
const events = require('events');
//Create an event emitter object
const eventEmitter = new events.EventEmitter();
//Create an event handler
const eventHandler = () => {
console.log('Event Received Successfully.');
}
//Attach event handler to an event
eventEmitter.on('event', eventHandler);
//Emit an event
eventEmitter.emit('event');
By following these steps, you can implement event-driven programming in Node.js effectively. Remember, the key to success in event-driven programming is proper event naming conventions and effective handling of events.
Benefits and Challenges of Event Driven Architecture
Event Driven Architecture (EDA) has gained popularity in modern software development due to its benefits in enabling decoupling, asynchronous processing, and scalability. However, as with any design pattern, it also presents its own set of challenges.
Benefits of Event Driven Architecture
One of the most significant benefits of EDA is decoupling, which allows for more flexible and modular application design. By separating components and services into independent modules, developers can more easily modify and update specific parts without affecting the entire system.
Another advantage of EDA is enabling asynchronous processing, which can improve performance and reduce latency. With asynchronous processing, applications can process multiple tasks simultaneously, improving response times and overall system efficiency.
Scalability is also a key benefit of EDA. By leveraging distributed systems and message queues, applications can scale horizontally, adding more instances as needed to handle increased load.
Challenges of Event Driven Architecture
One of the main challenges of EDA is the increased complexity it introduces. With the event-driven design pattern, components are loosely coupled, making it harder to follow the flow of control in the system. Additionally, with events potentially being processed out of order, proper error handling becomes critical to ensure data consistency.
Another challenge in EDA is ensuring that events are properly named and versioned. Inconsistent naming conventions or versioning practices can lead to confusion and errors, making it essential to establish clear standards and documentation.
Event Driven Architecture Best Practices
Implementing Event Driven Architecture (EDA) can be challenging, but following best practices can make it easier and more effective. Here are some tips for designing and implementing EDA in your Node.js projects:
1. Use Clear and Consistent Event Naming Conventions
Choosing clear and consistent event names is essential for creating a scalable and maintainable event-driven system. Use descriptive names that accurately reflect the event’s purpose, and use a consistent format to ensure the events are easy to understand and use across the system.
2. Version Your Events
Versioning your events can help you manage changes to your event-driven system over time. By specifying a version number for each event, you can ensure that changes to the events are backward-compatible and that the system can evolve without disrupting existing workflows.
3. Implement Proper Error Handling
Effective error handling is crucial for maintaining the integrity and reliability of your event-driven system. Make sure to handle errors appropriately at every stage of the event lifecycle, from emitting events to handling them in the system. Use error codes and messages to help diagnose and resolve issues quickly.
4. Test Your Event-Driven System Thoroughly
Thorough testing is essential for identifying and addressing issues in your event-driven system before they impact users. Use automated testing tools and techniques to test every aspect of the system, including event emission, handling, and data consistency.
5. Ensure Your Event-Driven System is Asynchronous
Asynchronous processing is a core principle of event-driven systems. Make sure that your system is designed to handle events asynchronously, allowing it to scale as the workload increases and avoiding performance bottlenecks.
By following these best practices, you can ensure that your event-driven system is efficient, scalable, and maintainable. Keep exploring the world of event-driven architecture in Node.js and don’t forget to use these best practices to ensure the success of your projects.
Real-World Use Cases of Event Driven Architecture in Node js
Event Driven Architecture is a powerful design pattern that has been adopted across various industries and use cases. Let’s take a look at some real-world examples of how Node.js Event Driven Architecture has been implemented to solve complex problems and create scalable applications.
Use Case 1: E-commerce platform
An e-commerce platform implemented an event-driven system in Node.js to manage its inventory and order system. By using event-driven communication between services, the platform was able to process orders faster and with fewer errors. The inventory service emits a “product sold” event, which triggers the order service to create an order and update the inventory. This architecture reduced latency and ensured consistent data integrity across all services.
Use Case 2: Social Media Platform
A social media platform used Event Driven Architecture to power its real-time notification system. By using an event-driven system, the platform was able to notify users of updates to their feeds and interactions with their content in real-time. The notification service would emit events to update the user’s feed, profile view, and also send push notifications to their mobile devices. The event-driven system ensured that notifications were delivered reliably and quickly, improving user experience and engagement drastically.
Use Case 3: Healthcare platform
A healthcare platform implemented Event Sourcing with Node.js to maintain data consistency and track patient records. By using an event-driven approach, they were able to track every change to a patient record and view the history of changes made to the record. If a change was made incorrectly, the platform could roll back the changes to a previous state accurately. This architecture offered a complete audit trail of patient records and ensured data consistency across multiple services.
These are just a few examples of how Node.js Event Driven Architecture can be implemented to improve scalability, data consistency, and reliability. By leveraging the power of events in a Microservices architecture, developers can create more efficient, scalable, and reliable applications with minimal latency and maximum uptime.
Conclusion
Congratulations, you have reached the end of our comprehensive guide on mastering Event Driven Architecture in Node js! We hope that this guide has provided you with valuable insights into the world of event-driven programming and architecture.
As we have seen, EDA offers several benefits such as scalability, decoupling, and asynchronous processing, making it a powerful tool for modern software development.
Remember, Event Driven Architecture is not a one-size-fits-all solution and may not always be the best approach for your application. However, it is a valuable design pattern to have in your toolbox and can greatly improve your application’s performance and scalability when used appropriately.
We encourage you to continue exploring and experimenting with Event Driven Architecture in your Node.js projects. As you gain more experience, you will become more proficient with event-driven programming and architecture concepts.
Thank you for taking the time to read our guide. Happy coding with events!
FAQ
What is Event Driven Architecture?
Event Driven Architecture (EDA) is a design pattern that allows software systems to respond to events and messages, rather than being driven by a predefined sequence of actions. In EDA, components communicate with each other through events, enabling loose coupling and asynchronous processing.
Why is Event Driven Architecture important in Node.js?
Event Driven Architecture is particularly relevant in Node.js due to its non-blocking, event-driven nature. Node.js excels at handling multiple concurrent connections and processing events efficiently, making it an ideal platform for building scalable and highly responsive applications.
What are the benefits of Event Driven Architecture?
Event Driven Architecture offers several benefits, including improved scalability, modularity, and flexibility. It enables asynchronous processing, allowing systems to handle a large number of concurrent events without blocking or slowing down. Additionally, EDA promotes loose coupling between components, making applications easier to maintain, test, and extend.
How can I implement Event Driven Architecture in my Node.js application?
To implement Event Driven Architecture in Node js, you can use the built-in EventEmitter module or any other event-driven libraries. Start by identifying the events that your application needs to handle and define event listeners to respond to those events. Emit the events when appropriate, and handle them asynchronously using event-driven workflows.
Are there any best practices for designing Event Driven Architecture?
Yes, there are several best practices for designing Event Driven Architecture in Node js. Some key practices include using descriptive and semantically meaningful event names, versioning events to ensure backward compatibility, handling errors gracefully, and thoroughly testing event-driven workflows to ensure reliability and maintainability.
What are some real-world use cases of Event Driven Architecture in Node js?
Event Driven Architecture has been successfully implemented in various industries and applications. Some examples include real-time collaboration platforms, IoT systems, financial trading systems, and chat applications. EDA enables these systems to handle a large number of concurrent events and provide real-time updates to users.
Next tutorial: Step By Step Guide to Setup Node js Environment Successfully
1 Comment