Welcome to this article on Context API in React js, where we will be discussing how this feature can enhance the performance of your web development projects. Context API is a powerful tool that enables efficient data handling by facilitating state management in React js applications. By utilizing the Context API, developers can streamline their code and make their applications more maintainable.
Context API is an essential feature of React js that allows developers to manage the state of their application across different components. It simplifies the process of data handling by eliminating the need to pass data between components with props, also known as prop drilling. By creating contexts, information can be shared and accessed within the same component tree, making data management more efficient.
In the following sections, we will delve deeper into the topic of Context API in React js, exploring its core concepts, advantages, and best practices for implementation. We will also discuss the need for Context API and compare it to other approaches for state management in React js applications.
Join us as we explore the potential of Context API in React js and discover how this feature can help you optimize your web development projects.
Understanding State Management in React Js
State management is a critical concept in React Js that enables efficient data handling in web development projects. At its core, state management allows developers to manage and update component data dynamically, making it an essential tool for creating interactive user interfaces.
With state management in React Js, you can easily manipulate individual elements of an application’s user interface, such as changing the text in a button or updating the value of an input field. By updating the state of a specific component, you can refresh its appearance and enable user interactions without having to reload the entire page.
The Role of State in React Js
In React Js, state refers to the current data captured by a component. Unlike props, which are passed down from parent components to children, state is managed by the component itself. By defining state, developers can create dynamic and interactive user interfaces that respond to user inputs and other changes in the application’s data.
Using state management in React Js, developers can retain data across multiple user interactions and updates, making it possible to create personalized user experiences that respond in real-time to user actions.
Tools for Managing State in React Js
React Js provides several tools for managing state, including hooks like useState and useContext. These tools enable developers to create components with self-contained state data that can be updated and managed independently.
By using hooks like useState, developers can easily create and update component state data without having to write complex code. useContext, on the other hand, enables developers to share state data across multiple components, making it an invaluable tool for managing data “globally” across an application.
Benefits of State Management in React Js
State management is a fundamental concept in React Js that provides several benefits for web development projects. By allowing developers to manage and update component data dynamically, state management makes it possible to create interactive and engaging user experiences that respond in real-time to user inputs.
State management in React Js also enables more efficient code reuse and reduces the need for complex data flow architectures, making the development process faster and more streamlined. Additionally, by using state management tools like useContext, developers can significantly reduce the complexity of code and minimize the risk of errors or bugs arising from data handling issues.
- SEO keywords: State management, React Js
The Need for Context API
As web developers, we know how crucial it is to manage and share data across different components in our React Js applications. Without proper data handling, our code can quickly become cluttered and difficult to maintain. Here is where Context API comes into play.
Context API is a way to manage global state in React Js applications, enabling us to pass data down through the component tree without having to rely on props. This approach can significantly simplify our code and make it more readable, ultimately leading to improved efficiency and better performance.
Moreover, Context API also allows us to update and maintain our data effortlessly, eliminating the need for excessive refactoring and reducing the risk of bugs and errors in our code. With Context API, data handling in React Js becomes a breeze.
Core Concepts of Context API
When working with Context API in React Js, there are three core concepts that developers need to understand: providers, consumers, and the context object.
Providers
Providers are components that define the context and make it available to other components in the application. They use the createContext()
method from the React library to create a context object that can be passed to other components through their props.
Example:
<MyContext.Provider value={{data: this.state.data, updateData: this.updateData}}>
{this.props.children}
</MyContext.Provider>
In this example, MyContext.Provider
is the provider component that defines the context and passes it down to its children components. The context object has two properties: data
and updateData
.
Consumers
Consumers are components that access the context data and use it to render their content. They use the useContext()
hook to retrieve the context object from the provider and access its properties.
Example:
const myContext = useContext(MyContext);
return <div>{myContext.data}</div>;
In this example, myContext
is a variable that holds the context data retrieved from the MyContext
provider. The code then renders the value of the data
property from the context object.
The Context Object
The context object is the central piece of Context API that holds the data that need to be shared across components. It can hold any type of data, such as strings, numbers, objects, or arrays.
Example:
const MyContext = createContext({
data: {},
updateData: () => {}
});
In this example, the context object is created using the createContext()
method and is initialized with an empty object for the data
property and an empty function for the updateData
property. These properties can be updated later on when the context is used.
By understanding these core concepts of Context API in React Js, developers can create efficient and scalable applications that handle data in a streamlined and organized way.
Implementing Context API in React Js
Implementing Context API in a React Js project is a straightforward process and can greatly improve the efficiency of data handling within your application. Here are the steps to follow:
Create a Context Object
The first step is to create a context object that will hold the data to be shared between components. This is typically done in a separate file to keep the code organized. To create a context object, use the createContext() method in the React library. Here’s an example:
const MyContext = React.createContext();
Create a Provider Component
Next, create a Provider component that wraps around the components that will receive the shared data. The Provider component will pass the data down to the child components through props. Here’s an example:
import React, { createContext, useState } from 'react';
const MyContext = createContext();
function MyProvider(props) {
const [state, setState] = useState("Hello World");
return (
<MyContext.Provider value={[state, setState]}>
{props.children}
</MyContext.Provider>
);
}
In this example, the Provider component is called MyProvider and it holds the string “Hello World” in its state. The context object is passed as a value to the Provider component using the value prop, with the state and setState functions wrapped in an array.
Create a Consumer Component
Finally, create a Consumer component that receives the shared data from the Provider component. The Consumer component will use the useContext() hook to access the data from the context object. Here’s an example:
import React, { useContext } from 'react';
const MyConsumer = () => {
const [state, setState] = useContext(MyContext);
return (
<div>
{state}
</div>
);
}
export default MyConsumer;
In this example, the Consumer component is called MyConsumer and it uses the useContext() hook to access the shared data from the MyContext object. The state and setState functions are destructured from the array and can be used to update the shared data.
By following these steps, you can easily create and use contexts to handle shared data across components in your React Js applications.
Advantages of Context API
Using Context API in React Js offers several advantages over other state management techniques. Let’s take a look at some of the key benefits:
1. Simpler Prop Drilling
Prop drilling refers to the process of passing props down through multiple levels of components to reach a specific component that needs the data. Context API simplifies this process by allowing data to be accessed directly from any component in the hierarchy without the need for intermediate components to pass it down.
2. Improved Code Readability
With Context API, your code becomes more readable as it eliminates the need for complex prop passing between components. This makes the code easier to understand and maintain.
3. Easy Maintenance
Context API provides a centralized location for data management. This makes it easier to maintain and update as changes can be made in one place, rather than updating multiple components throughout the application.
4. Increased Performance
Context API improves the performance of React Js applications as it reduces the number of renders required to update the state of the application. By providing a centralized location for data management, unnecessary renders can be avoided, leading to a faster and more efficient application.
Overall, using Context API in your React Js projects can simplify data management, improve code readability and maintainability, and boost performance. It is a powerful tool that can help you build more robust and efficient web applications.
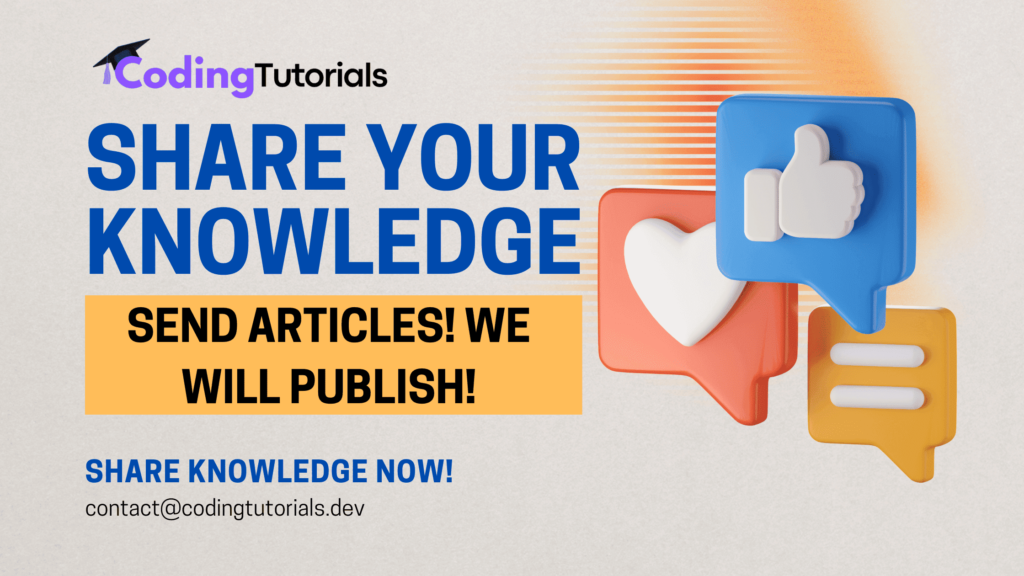
Using Multiple Contexts
In React Js, managing data across multiple components can be a challenging task when using props. Context API makes the process easier and more efficient by allowing developers to create and use multiple contexts. This approach is particularly useful when dealing with different types of data, such as user authentication and theme settings.
When working with multiple contexts in Context API, it is essential to maintain a clear structure and avoid nesting contexts inside each other. Each context should have its own provider and consumer components so that the data flow remains organized and readable.
For example, imagine a React Js application that has multiple components that require theme settings and user authentication data. By using separate contexts for each type of data, developers can avoid confusion and simplify their code.
Benefits of Using Multiple Contexts
- Reduces complexity: Using multiple contexts can help to avoid unnecessary complexity and simplify the data flow in React Js applications.
- Improves performance: By using separate contexts, developers can ensure that the data is only passed to the relevant components, reducing the number of unnecessary re-renders and improving application performance.
- Enables modularity: Multiple contexts enable developers to create modularity in their code, making it easier to maintain and update the application over time.
Overall, using multiple contexts in Context API is a powerful technique for managing and sharing data across components in React Js applications. By maintaining a clear structure and following best practices, developers can take full advantage of this approach and build high-quality, efficient applications.
Best Practices for Context API
Context API is a powerful tool for state management in React Js projects. To ensure optimized performance and maintainable code, it’s important to follow best practices when implementing Context API. Here are some guidelines for using Context API effectively:
1. Use Context API Sparingly
While Context API can be useful for managing and sharing data across components, it’s important not to overuse it. Too many contexts can make your code more complex and difficult to maintain. Consider whether using props or other state management tools might be a better fit for certain parts of your application.
2. Keep Context Objects Small
Context objects can quickly become bloated with unnecessary data. To keep your code clean and efficient, only include the data that is absolutely necessary for each context object.
3. Make Context Consumers Explicit
When using Context API, it’s important to make the consumer components explicit. This makes it easier to track where data is being used and can help prevent unexpected changes to your application’s state.
4. Use PureComponent or useMemo for Performance Optimization
For better performance, consider using PureComponent or useMemo when creating components that rely on context data. This can help prevent unnecessary re-renders and improve the overall speed of your application.
5. Avoid Using Context API for Global State
While Context API can be tempting for managing global state, it’s generally not the recommended approach. Instead, consider using a state management library like Redux or MobX.
6. Test Your Context API Implementation Thoroughly
As with any code, it’s important to thoroughly test your implementation of Context API. Use unit tests to ensure that your contexts and consumers are working correctly and that your application’s state is being managed effectively.
By following these best practices, you can use Context API to its fullest potential and achieve efficient state management in your React Js projects.
Alternatives to Context API
While Context API is a powerful tool for managing state in React Js, there are also alternative approaches that developers can consider. The most common alternatives are:
Redux
Redux is a popular tool for managing state in React Js applications. It provides a centralized store that can be accessed by any component and offers powerful features such as time-travel debugging and middleware.
MobX
MobX is another state management library that can be used in React Js applications. It uses observables to track changes and ensure that components are efficiently updated when data changes. It also provides a simple API that is easy to learn and use.
Prop Drilling
Prop drilling is a technique where data is passed down through multiple levels of components as props. While it can be effective in some cases, it can also make code more complex and difficult to maintain, especially in larger applications.
Each of these alternatives has its own advantages and disadvantages, and the best choice will depend on the specific needs of the project. However, it is worth noting that Context API is a built-in feature of React Js and can be a more lightweight and straightforward option for simpler projects.
Ultimately, the decision to use Context API or an alternative approach will depend on the developer’s preferences and the requirements of the project at hand.
Conclusion
In conclusion, Context API in React Js is a powerful tool that offers developers a more efficient way of handling data and managing state in their web development projects. By using Context API, developers can reduce the complexity of their code, improve performance, and enhance the maintainability of their applications.
While alternative approaches to state management exist, Context API stands out as a solution that is native to React Js and offers a simpler, more streamlined way of handling data. As such, it is important for developers to consider implementing Context API in their React Js projects.
Overall, the benefits of using Context API in React Js are clear. By applying the core concepts and best practices outlined in this article, developers can harness the full potential of this powerful tool and take their web development projects to the next level.
FAQ
What is the Context API?
The Context API is a feature in React Js that allows data to be passed down through the component tree without manually passing props at each level. It provides a way to share data between components without the need for prop drilling.
Why is state management important in React Js?
State management is crucial in React Js because it allows components to hold and manage their own data. It enables efficient data handling and ensures that changes in the data trigger component updates, leading to a more responsive user interface.
Why do I need the Context API?
The Context API is useful for managing and sharing data that needs to be accessed by multiple components. It eliminates the need for passing props through intermediate components, making data management more efficient and reducing code complexity.
What are the core concepts of the Context API?
The core concepts of the Context API are providers, consumers, and the context object. Providers wrap the components that need to access the shared data, consumers consume the data from the provider, and the context object holds the shared data.
How can I implement the Context API in React Js?
To implement the Context API in React Js, you need to create a context using the createContext function, wrap your components in a provider that supplies the context data, and access the context data in consuming components using the useContext hook or the Context.Consumer component.
What are the advantages of using the Context API?
Using the Context API in React Js has several advantages. It reduces the complexity of prop drilling, improves code readability by separating concerns, and makes it easier to share data between components without relying on a centralized state management library.
How can I use multiple contexts in React Js applications?
To use multiple contexts in React Js applications, you can nest the providers of different contexts, allowing components to access multiple sets of shared data. This approach is useful when managing different types of data that are unrelated to each other.
What are the best practices for using the Context API?
When using the Context API, it is recommended to avoid deeply nested contexts, as it can make the code harder to understand. It’s also important to consider the performance implications of using the Context API and optimize the data flow if necessary.
Are there any alternatives to the Context API for state management in React Js?
Yes, there are alternative approaches to state management in React Js, such as using third-party libraries like Redux or MobX. These libraries provide more advanced state management capabilities and can be suitable for larger, more complex applications.
Next Tutorial: Mastering the Node Js Express Framework: Your Guide to Server-Side JavaScript
1 Comment