If you’re a web developer, chances are you’ve come across the terms “Web APIs” and “Fetch API” in Javascript. Fetch API and Web APIs in javascript are essential tools that can help enhance your web development projects. In this guide, we’ll provide an overview of what Web APIs and the Fetch API are, and how they work together to enable efficient communication between different software systems.
At a high level, a Web API is a set of protocols and tools for building software applications. It defines how different software systems can interact with each other over the internet, allowing developers to integrate external functionality into their own applications. On the other hand, the Fetch API is a modern interface for making HTTP requests in Javascript. It simplifies the process of making API requests and handling responses, making it an essential part of any modern web developer’s toolkit.
In the upcoming sections, we’ll delve deeper into the functionality and usage of both Web APIs and the Fetch API. We’ll explain how you can use them to retrieve and manipulate data, how to handle authentication and authorization, and how to test and debug API requests. Whether you’re a seasoned developer or just starting, by the end of this guide, you’ll have a comprehensive understanding of Web APIs and the Fetch API in Javascript, and how you can leverage them to enhance your development projects.
Fetch API and Web APIs in Javascript: What are Web APIs?
If you’re new to the world of web development, you may be wondering what Web APIs are and why they matter. In short, Web APIs (Application Programming Interfaces) are a set of protocols, tools, and routines for building software applications. They enable communication between different software systems, allowing them to exchange data and interact with one another in a standardized way.
There are many different types of Web APIs, including those for social media, weather, maps, and payment processing. These APIs provide developers with access to a wealth of data and functionality that they can integrate into their applications, saving them time and effort.
How do they work?
Web APIs work by providing a standardized interface that can be accessed by other software systems. This interface is typically implemented using a set of rules and protocols, including HTTP (HyperText Transfer Protocol), REST (Representational State Transfer), and JSON (JavaScript Object Notation).
When a developer wants to use an API, they typically send a request to the API endpoint using a specific HTTP method (such as GET or POST), along with any required parameters or data. The API then processes the request and returns a response in a specific format, such as JSON or XML (eXtensible Markup Language).
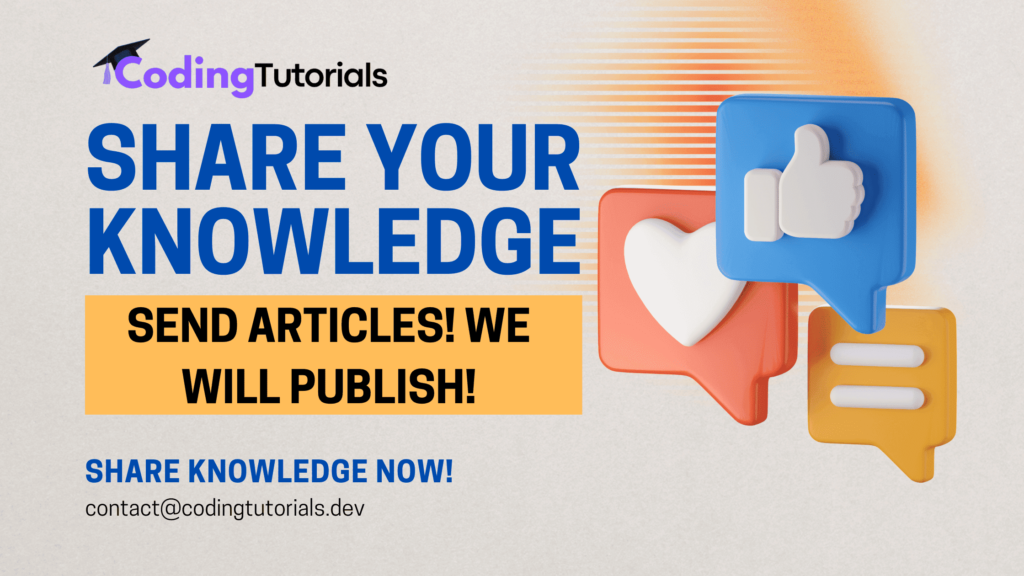
Why are they important?
Web APIs are an essential part of modern web development, as they allow developers to integrate third-party data and functionality into their applications easily. This, in turn, enables them to create more robust and feature-rich applications while saving time and effort.
Web APIs are also crucial for enabling communication between different software systems, which is becoming increasingly important as more applications and services become interconnected.
Example Use Case
Web API | Description |
---|---|
OpenWeatherMap API | A free to use API that provides real-time weather data for any location. |
Twitter API | An API that provides access to data related to Twitter, including tweets, user profiles, and trends. |
Stripe API | An API that enables developers to integrate payment processing into their applications easily. |
Web APIs are a crucial component of modern web development, enabling developers to create feature-rich applications that integrate seamlessly with other software systems. Stay tuned for the next section, where we’ll explore the Fetch API in more detail.
Exploring the Fetch API
In this section, we’ll take a closer look at the Fetch API and explore its features and benefits. The Fetch API is a modern interface for making HTTP requests in Javascript. It provides a cleaner and more flexible alternative to traditional AJAX requests, and also supports new functionality such as request cancellation and timeout handling.
The Fetch API is built on the Promise API, which allows for more readable and maintainable code. It also supports streaming requests and responses, making it ideal for handling large files or responses that are too large to fit entirely in memory.
One of the key differences between the Fetch API and traditional AJAX requests is the way in which requests and responses are handled. With the Fetch API, requests and responses are treated as streams of data, which can be processed as they arrive. This allows for more efficient and optimized data handling, particularly for large data sets.
Another unique feature of the Fetch API is the ability to easily customize and configure requests using options such as headers, mode, credentials, and cache. These options enable developers to fine-tune their requests and ensure that they are secure, efficient, and compliant with any relevant regulations or standards.
Overall, the Fetch API is a powerful and versatile tool for making HTTP requests in Javascript. Its modern design and flexible functionality make it an ideal choice for web developers looking to enhance the performance and functionality of their web applications.
Using the Fetch API for GET Requests
The Fetch API provides a simple and efficient way to make GET requests to external APIs. In this section, we’ll explore the syntax and functionality of using the Fetch API for GET requests.
Fetch API GET Syntax
Making a GET request with the Fetch API involves using the fetch() method and passing in the URL of the API endpoint you wish to access:
fetch('https://example-api.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Error fetching data: ", error));
The above code example shows how to fetch data from an API and handle the response using the Promise-based syntax of the Fetch API. It makes a GET request to the URL https://example-api.com/data and logs the response data to the console. The .json() method is used to extract the JSON data from the response.
Handling Responses
The response object returned by the Fetch API contains metadata about the response, such as the status code and headers, as well as the actual response data. It’s important to handle the response appropriately based on the status code and expected response format. For example, if the response status is 404 (Not Found), you may want to display an error message to the user.
The code below demonstrates how to handle different response status codes:
fetch('https://example-api.com/data')
.then(response => {
if (response.status === 404) {
console.error("Error: 404 Not Found");
} else {
return response.json();
}
})
.then(data => console.log(data))
.catch(error => console.error("Error fetching data: ", error));
Retrieving Data from External APIs
After making a GET request with the Fetch API, you can retrieve specific data from the response using the dot notation. For example, if the response data contains a “name” field, you can access it like this:
fetch('https://example-api.com/data')
.then(response => response.json())
.then(data => console.log(data.name))
.catch(error => console.error("Error fetching data: ", error));
The Fetch API also allows you to pass additional parameters to the API endpoint, such as query parameters, to retrieve more specific data. For example, to retrieve only data with a specific ID:
fetch('https://example-api.com/data?id=123')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Error fetching data: ", error));
Making POST Requests with the Fetch API
In the previous section, we learned how to use the Fetch API for GET requests. Now, we’ll explore how to make POST requests using the same API. POST requests are typically used to submit data to a server, such as when submitting a contact form or creating a new user account.
The syntax for a basic POST request with the Fetch API looks like this:
fetch(url, {
method: 'POST',
body: JSON.stringify(data)
})
Sending Data to Servers
When making POST requests, it’s essential to send data to the server in the correct format. The Fetch API requires data to be in the form of a string, so we must convert the data to a JSON string using the JSON.stringify() method.
For example, let’s say we want to submit a form with the user’s name and email address. Here’s how we can create the data object and send it as part of the POST request:
const form = document.querySelector('#contact-form');
const data = {
name: form.name.value,
email: form.email.value
};
fetch('/api/contact', {
method: 'POST',
body: JSON.stringify(data)
})
The above code creates a new data object with the values of the name and email input fields in a contact form. It then sends a POST request to the server at the /api/contact endpoint, including the data as a JSON string in the body of the request.
Handling Response Codes
Just like with GET requests, the server can respond to a POST request with a variety of response codes. These codes indicate the success or failure of the request and can provide additional information about any errors that occurred.
Here are some common response codes you may encounter when making POST requests:
Response Code | Description |
---|---|
200 OK | The request was successful |
201 Created | The resource was successfully created |
400 Bad Request | The server could not understand the request data |
401 Unauthorized | The request requires user authentication |
404 Not Found | The requested resource was not found on the server |
500 Internal Server Error | The server encountered an unexpected error |
It’s important to handle response codes appropriately when making POST requests. Depending on the code, you may need to display an error message to the user or take other actions to correct the issue.
Dealing with Errors
As with any web development project, errors can occur when making POST requests. These errors can be caused by a variety of factors, such as server downtime, network latency, or incorrect request data.
The Fetch API provides a catch() method that allows you to catch and handle any errors that occur during the request. For example:
fetch('/api/contact', {
method: 'POST',
body: JSON.stringify(data)
})
.then(response => {
if (response.ok) {
return response.json();
} else {
throw new Error('Network response was not OK');
}
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
The above code attempts to make a POST request to the /api/contact endpoint and handle the response data. If the server returns a successful response, the data is logged to the console. If the server returns an error response, the catch() method logs the error to the console.
By using the catch() method, we can gracefully handle errors that may occur during the request and provide feedback to the user.
Working with JSON Data
JSON, short for JavaScript Object Notation, is a lightweight format for storing and exchanging data. JSON is widely used in Web APIs as a data format, and the Fetch API enables easy integration of JSON data into your Javascript applications.
When working with JSON data, it’s important to ensure that the data is properly formatted and parsed. This can be achieved using the built-in JSON.parse() and JSON.stringify() methods in Javascript.
The JSON.parse() method is used to convert a JSON string into a Javascript object. For example:
const jsonStr = '{"name":"John Doe","age":30}';
const jsonObj = JSON.parse(jsonStr);
console.log(jsonObj.name); // Output: John Doe
console.log(jsonObj.age); // Output: 30
The JSON.stringify() method does the opposite, converting a Javascript object into a JSON string. For example:
const jsonObj = {
"name": "John Doe",
"age": 30
};
const jsonStr = JSON.stringify(jsonObj);
console.log(jsonStr); // Output: {"name":"John Doe","age":30}
When working with JSON data in the Fetch API, it’s important to specify the Content-Type header as application/json to ensure that the server knows to expect JSON data in the request body. For example:
fetch('https://api.example.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
name: 'John Doe',
age: 30
})
});
The Fetch API can also easily retrieve JSON data from external APIs. For example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
});
By utilizing JSON data and the Fetch API, you can create powerful, dynamic web applications that can easily integrate with various Web APIs.
Authentication and Authorization with Web APIs
When working with Web APIs, it is crucial to ensure that the API endpoints are protected from unauthorized access. Authentication and authorization are two essential concepts that can help secure your Web API. Authentication is the process of verifying the identity of a user or a client that is trying to access the API endpoint. Authorization, on the other hand, is the process of determining whether the authenticated user or client has permission to perform a specific action on the API endpoint.
Types of Authentication
There are several types of authentication that you can implement in your Web API. One common type is the API Key authentication. With this method, you generate a unique API key for each client that wants to access your API. The client must include the API key in each request to the API endpoint to authenticate itself.
Another popular authentication method is Token-based authentication. With this method, the client first sends its credentials to the server, and if they are valid, the server returns a token. The client then includes this token in each subsequent request to the API endpoint to authenticate itself. The server can validate the token to identify the client and determine whether it has the necessary permissions to perform the requested action.
Authorization with Web APIs
Authorization can be more complex than authentication because it involves determining whether a specific user or client has permission to perform a specific action on the API endpoint. One common approach to authorization is using roles and permissions. With this method, you define roles such as ‘Admin’, ‘User’, or ‘Guest’ and assign specific permissions to each role. For instance, an ‘Admin’ role might have permission to perform all actions on the API endpoint, while a ‘User’ role might only have permission to perform specific actions.
Best Practices for Authentication and Authorization
When implementing authentication and authorization in your Web API, there are several best practices that you should follow:
- Use HTTPS to encrypt the communication between the client and the API endpoint to prevent eavesdropping and tampering.
- Keep your API keys and tokens secure by not storing them in plain text and limiting their exposure.
- Implement rate limiting to prevent abuse and lower the risk of denial-of-service attacks.
- Regularly review and audit your authentication and authorization mechanisms to identify and address vulnerabilities.
By following these best practices, you can ensure that your Web API is secure and protected from unauthorized access.
Handling Errors and Error Handling with Fetch API
When working with Web APIs and the Fetch API in Javascript, it’s essential to be prepared to handle errors effectively. Errors can occur in the network, server, or client-side, and knowing how to identify and resolve them is crucial to maintaining the reliability and functionality of your application.
The Fetch API provides several mechanisms for handling errors and running error-handling code in case of issues. In this section, we’ll explore some common error scenarios and detail how to handle them effectively using the Fetch API.
Error scenarios
Some common error scenarios to look out for when working with Fetch API requests include:
- Network errors- network connection lost, server down
- Invalid URLs- incorrect URLs or URLs that do not exist
- Request errors- syntax or runtime errors in the request code
- Server errors- backend status codes indicating server-side issues
Error handling with Fetch API
Here are some of the key ways to handle errors when working with the Fetch API:
- Use try-catch blocks to catch exceptions thrown during the Fetch API call.
- Check the response object to verify whether the request was successful or not. If the response status code is within the 200-299 range, the request is considered successful. Otherwise, an error has occurred.
- Use Promise-based syntax to handle errors gracefully. This allows you to run code that handles specific error scenarios and provides a fallback mechanism for when errors occur.
- Handle network errors by catching exceptions thrown by the fetch() function, as well as by using the catch() method of the Promise returned by the fetch() function.
Example of Fetch API error handling
Here’s an example of using the Fetch API to handle errors:
Handling network errors
try {
const response = await fetch('https://example.com/data.json');
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
} catch (error) {
console.error('Error:', error);
}
Handling invalid URLs
try {
const response = await fetch('https://example.com/some-invalid-url');
if (!response.ok) {
throw new Error('Invalid URL');
}
const data = await response.json();
} catch (error) {
console.error('Error:', error);
}
Handling server errors
try {
const response = await fetch('https://example.com/data.json');
if (!response.ok) {
throw new Error('Server error');
}
const data = await response.json();
} catch (error) {
console.error('Error:', error);
}
By following these practices, you can ensure that your application remains stable and performs optimally in the event of errors. Remember, handling errors effectively is just as important as preventing them.
Integrating Web APIs into Javascript Applications
Web APIs can enhance your Javascript applications by providing access to external data and functionality. Integrating Web APIs into your application can improve its performance, increase its functionality, and make it more interactive. Here are some key considerations when integrating Web APIs into your Javascript applications:
Identify the Right Web API
Before implementing a Web API, it’s essential to determine which API is best suited for your application’s needs. There are numerous Web APIs available, each with its own specific functions and features. Hence, do thorough research and choose an API that aligns with your application’s goals and objectives, and has the features required to meet them.
Optimize API Calls
When integrating Web APIs, it’s essential to optimize API calls to improve your application’s performance. Too many API requests can cause slow page loading times and even crash your application. Hence, make sure to organize your API calls effectively, eliminate unnecessary requests, and use caching and request throttling to reduce the impact of too many requests.
Secure Your API calls
API security is crucial to protecting the data transmitted between your application and the API server. Hence, ensure you use HTTPS protocols, implement user authentication, and use encryption to protect your API calls from man-in-the-middle attacks. API keys, rate limiting, and token-based authentication are also effective methods of securing your API calls.
Test and Debug Your Integration
Integrating Web APIs into your Javascript application can be complex, and unexpected errors may arise. Hence, it’s essential to test and debug your Web API integration to ensure its reliability and functionality. Tools like Postman and SoapUi can help test your API integration, while browser dev tools can help diagnose and troubleshoot unexpected errors.
Integrating Web APIs into your Javascript application can provide significant benefits. By following the above considerations, you can ensure a successful integration, resulting in an application that’s more interactive, efficient, and functional.
Cross-Origin Resource Sharing (CORS) and Fetch API
When making API requests from different domains, you may encounter Cross-Origin Resource Sharing (CORS) issues. CORS is a security feature implemented by browsers to restrict web pages from making requests to a different domain than the one that served the web page.
The Fetch API provides a way to handle CORS-related issues by allowing you to specify the mode of the request. The mode determines the restrictions enforced by the browser when sending the request.
CORS Modes
There are three modes for CORS requests:
- no-cors: This mode is used when making requests to a different domain, but you don’t need to access the response’s data. It only allows simple GET/POST requests.
- cors: This mode is the default mode for requests within the same origin. In this mode, the browser will include an Origin header in the request to check if the server can allow the request from a different domain.
- same-origin: This mode only allows requests to be made within the same origin, and it cannot access data from different domains.
Handling CORS Errors
To handle CORS errors, you can use try…catch blocks to catch any exceptions that may occur. You can also use the .catch() method to handle errors when making Fetch API requests.
Additionally, if you control the server, you can configure it to allow requests from other domains by setting appropriate Access-Control headers.
In conclusion, understanding how to handle CORS-related issues when using the Fetch API is crucial for successful API communication. By specifying the correct mode and handling errors effectively, you can ensure that your API requests are reliable and secure.
Testing and Debugging Fetch API Requests
Testing and debugging are essential for ensuring the reliability and functionality of Fetch API requests. Here are some tips and tools to help you effectively test and debug your API requests:
1. Console.log
You can use console.log to display information in the console. This is useful for debugging code and checking the values of variables. You can use console.log to output the response data or any errors that occur:
fetch('https://example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
2. Network Tab
The network tab in your browser’s developer tools can be used to inspect the HTTP requests and responses made by your application. This can help you identify any errors or issues with your requests:
Method | URL | Status |
---|---|---|
GET | https://example.com/data | 200 OK |
POST | https://example.com/submit | 404 Not Found |
3. Postman
Postman is a popular tool for testing APIs. You can use it to make requests to your API and inspect the responses. Postman also provides a wide range of features, such as authentication and request history:
- Create a new Request
- Enter the URL for your request
- Select the appropriate HTTP method (GET, POST, etc.)
- Specify any request parameters or headers
- Click “Send” to make the request
4. Unit Testing
You can use unit tests to check the functionality of your code in isolation. This can be done using a testing framework such as Jest or Mocha. Unit tests can help you identify issues early on and prevent regressions:
test('successful request', async () => {
const response = await fetch('https://example.com/data')
const data = await response.json()
expect(data).toEqual({ name: 'John', age: 30 })
})
test('error handling', async () => {
const response = await fetch('https://example.com/notfound')
expect(response.status).toEqual(404)
})
Conclusion
We hope this comprehensive guide on Web APIs and the Fetch API in Javascript has been useful to you. We’ve covered a lot of ground, including an overview of Web APIs, a detailed exploration of the Fetch API, and best practices for integrating APIs into your Javascript applications.
By now, you should have a good understanding of how these powerful tools can enhance your development projects, enable seamless communication between different software systems, and facilitate secure data transfer.
Keep Exploring and Applying What You’ve Learned
There is much more to learn and explore when it comes to Web APIs and the Fetch API in Javascript. We encourage you to continue your learning journey, experiment with these tools, and apply what you’ve learned to your future development projects.
Thank you for reading, and happy coding!
FAQ
What are Web APIs?
Web APIs, also known as Application Programming Interfaces, are sets of tools and protocols that allow different software systems to communicate and interact with each other over the internet. They enable developers to access and utilize functionalities of external services, such as retrieving data from databases or interacting with social media platforms.
What is the Fetch API?
The Fetch API is a modern interface in JavaScript that provides a way to make HTTP requests, such as GET, POST, PUT, DELETE, etc. It offers a more streamlined and flexible approach compared to traditional AJAX requests. With the Fetch API, developers can easily send and receive data from servers, handle responses, and perform various operations with the data.
How do I use the Fetch API for GET requests?
To use the Fetch API for GET requests, you can simply make a fetch() call to the desired URL. You can provide additional options, such as headers or query parameters, to customize the request. Once the request is sent, you can handle the response using promises and extract the data you need.
Can I make POST requests with the Fetch API?
Yes, the Fetch API allows you to make POST requests as well. You can pass in an additional options object that includes the method set to “POST” and the necessary data to be sent to the server. The Fetch API also provides methods for handling response codes and errors, making it convenient to work with POST requests.
How do I handle JSON data with the Fetch API?
JSON (JavaScript Object Notation) is commonly used for data exchange in Web APIs. With the Fetch API, you can easily handle JSON data by using the .json() method on the response object. This method parses the response body as JSON and returns a promise that resolves to the actual data, allowing you to work with JSON objects effortlessly.
What are some authentication and authorization methods I can implement with Web APIs?
There are various authentication and authorization methods you can implement with Web APIs, depending on your requirements. Some common approaches include using API keys, OAuth, JWT (JSON Web Tokens), or session-based authentication. These methods ensure that only authorized users can access certain resources or perform specific actions within your API.
How do I handle errors when working with the Fetch API?
When working with the Fetch API, you can handle errors by checking the response status code. For example, if the status code is in the 4xx or 5xx range, it indicates an error. You can use conditional statements to handle different error scenarios and take appropriate actions, such as displaying error messages or retrying the request.
How can I integrate Web APIs into my JavaScript applications?
Integrating Web APIs into JavaScript applications involves making API requests, handling responses, and incorporating the retrieved data into your application’s logic. You can utilize JavaScript frameworks, such as React or Angular, or use vanilla JavaScript to make AJAX calls or utilize the Fetch API. It’s important to consider the asynchronous nature of API requests and implement appropriate error handling and data processing mechanisms.
What is Cross-Origin Resource Sharing (CORS) and how does it relate to the Fetch API?
Cross-Origin Resource Sharing (CORS) is a security mechanism that browsers use to enforce restrictions on cross-origin requests. It prevents malicious code from accessing resources on different domains. When making API requests with the Fetch API, CORS can come into play if the request is being made from a different origin. You might need to handle CORS-related issues by configuring proper response headers on the server or using CORS proxies.
How can I test and debug Fetch API requests?
Testing and debugging Fetch API requests can be done using browser developer tools, such as the Chrome DevTools or Firefox Developer Tools. These tools allow you to inspect network requests, view request and response headers, and monitor data flow. Additionally, you can use third-party tools like Postman or cURL to test API endpoints and verify the responses.
Next tutorial: Mastering WebSockets and Real-Time Applications in JavaScript
Leave a Reply