Welcome to our comprehensive guide on functional programming with Javascript. In this guide, we’ll explore how to enhance your coding skills and build dynamic web apps seamlessly using functional programming concepts. Whether you’re a beginner or an experienced developer, this easy guide will help you get started with functional programming with Javascript.
Functional programming is a programming paradigm that emphasizes writing code using pure functions and immutable data. In functional programming, functions are treated as first-class citizens, enabling you to write more concise and modular code. Javascript, as a versatile programming language, has built-in support for functional programming concepts, making it an excellent choice for building powerful and dynamic web applications.
In this guide, we’ll cover everything you need to know about functional programming with Javascript. We’ll start with an introduction to functional programming, discussing the core principles and benefits of adopting a functional programming approach in your Javascript codebase. We’ll then cover the fundamental concepts of functional programming, including immutability, pure functions, higher-order functions, and recursion. We’ll then delve into various functional programming paradigms that can be applied in Javascript, such as declarative programming, composition, and lazy evaluation. We’ll also explore how to work with higher-order functions, implement pure functions and immutability effectively, and transform data and compose functions.
Furthermore, we’ll discuss different approaches to handling errors, and explore how to leverage promises and embrace a functional style of asynchronous programming, enabling you to write more reliable and maintainable asynchronous code. We’ll also discuss strategies and tools for effectively testing and debugging functional Javascript code.
By mastering functional programming concepts, you can enhance your coding skills, build dynamic web apps, and write cleaner and more maintainable code. Let’s dive into the world of functional programming with Javascript!
Introduction to Functional Programming
Welcome to our easy guide on functional programming with Javascript! In this section, we’ll provide a comprehensive introduction to functional programming, one of the most popular programming paradigms in modern web development.
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Instead, functional programming encourages the use of pure functions – functions that always return the same output given the same input, with no side-effects.
Functional programming has gained popularity over recent years, thanks to its ability to simplify code, reduce complexity, and make it easier to reason about. It’s also well-suited for parallel processing and asynchronous programming – two critical concepts in modern web development.
So, what makes functional programming so unique? Functional programming encourages the use of higher-order functions, closures, and recursion to manipulate data. It promotes immutability and favors declarative programming over imperative programming.
By adopting a functional programming approach, you can write cleaner, more maintainable code, with fewer bugs and greater reliability.
We’ll dive deeper into functional programming concepts and their applications in Javascript in the upcoming sections, so stay tuned!
The Fundamentals of Functional Programming
Functional programming is based on fundamental concepts that differ from traditional programming paradigms. Understanding these concepts can be tricky, but it’s essential to build a solid foundation for your code. In this section, we’ll cover the fundamental concepts of functional programming, including immutability, pure functions, higher-order functions, and recursion. Let’s dive in!
Immutability
In functional programming, immutability is a critical concept that emphasizes that once a value is defined, it cannot be changed. Instead of changing values, functional programming favors creating new values based on existing ones. Immutable data structures can help simplify your code and ensure it’s less error-prone. Here’s an example:
Traditional Programming | Functional Programming |
---|---|
x = x + 1 | y = x.map(i => i + 1) |
The above example showcases two ways to increment a variable. In traditional programming, you directly change the value of x. However, in functional programming, you create a new array y based on x and apply the map() function to increment each element of the array by one. This way, the original array x is not modified, and we create a new one y.
Pure Functions
Pure functions are functions that have no side effects and always return the same output for a given input. A pure function doesn’t modify any external variable or object, and its output is entirely dependent on its input. This predictability makes it easier to track down bugs and avoid unwanted surprises. Here’s an example:
function add(a, b) {
return a + b;
}
The function add() is an example of a pure function: it only depends on its arguments, and it always returns the same output for a given input. It doesn’t modify any external variables or objects and has no side effects.
Higher-Order Functions
Higher-order functions are functions that can be passed as arguments or returned as values from other functions. Higher-order functions help in creating composable functions, which can be combined to achieve more complex functionality. Here’s an example:
let multiplyBy = (n) => {
return (x) => {
return x * n;
};
}
let multiplyByTwo = multiplyBy(2);
let result = multiplyByTwo(5); // result = 10
The example above demonstrates a higher-order function multiplyBy(), which returns another function. This function multiplies its input by the number passed as an argument to multiplyBy(). By calling multiplyBy() with the argument 2, we create a new function multiplyByTwo(), which multiplies its input by 2. The result of calling multiplyByTwo() with the argument 5 is 10.
Recursion
Recursion is a technique of solving a problem by solving smaller instances of the same problem. In functional programming, recursion is a common way to implement iteration. Recursive functions are functions that call themselves until a base condition is met. Here’s an example:
function factorial(num) {
if (num === 0) {
return 1;
}
else {
return num * factorial(num - 1);
}
}
let result = factorial(5); // result = 120
The example above demonstrates a recursive function factorial(), which calculates the factorial of a number. The function calls itself until the base condition (num === 0) is met. In this case, the function returns 1. Otherwise, it multiplies the number by the factorial of num-1.
Understanding these fundamental concepts is crucial to writing efficient and clean functional code in Javascript. In the next section, we’ll explore how to apply these concepts in various functional programming paradigms.
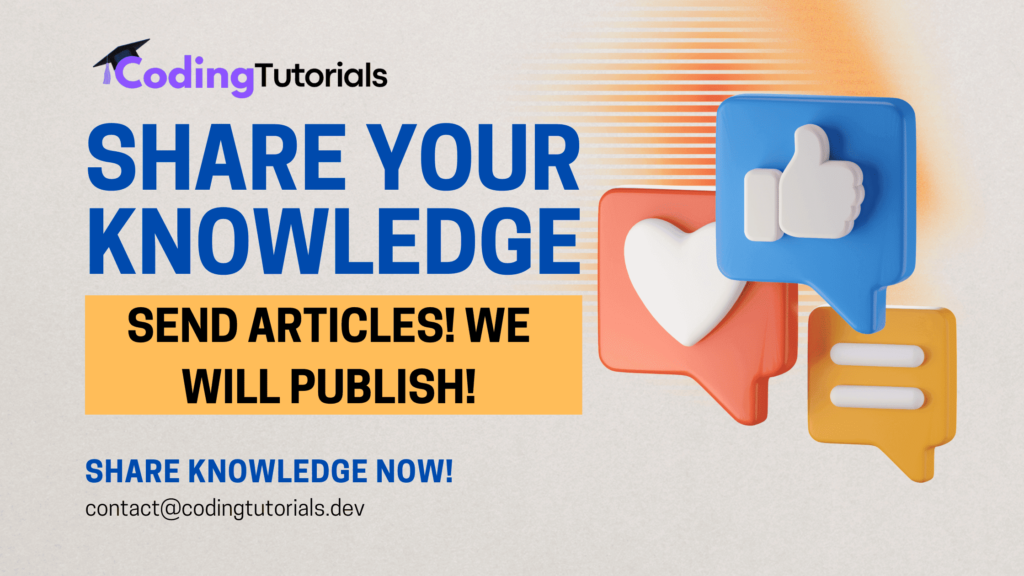
Functional Programming Paradigms in Javascript
Functional programming is a programming paradigm that emphasizes the use of pure functions and immutable data to build software. It provides several benefits such as improved code readability, maintainability, and testability. In this section, you’ll learn about various functional programming paradigms that can be applied in Javascript to build better software.
Declarative Programming
Declarative programming is a programming paradigm that expresses the logic of a computation without specifying its control flow. In other words, you describe what you want the program to do, but not how to do it. This paradigm is often used in functional programming and can be applied in Javascript using libraries like React and Redux.
Composition
Function composition is the process of combining two or more functions to produce a new function. It allows you to create complex functions by combining simpler functions. This paradigm can be applied in Javascript using libraries like Ramda and Lodash.
Lazy Evaluation
Lazy evaluation is a technique in functional programming where expressions are not evaluated until their values are needed. This technique can be used to improve performance by avoiding unnecessary computation. This paradigm can be applied in Javascript using libraries like RxJS and Lazy.js.
Comparison to OOP Paradigms
Functional programming paradigms differ from Object-Oriented Programming (OOP) paradigms. While OOP emphasizes the use of objects and their interactions to build software, functional programming defines computation as the evaluation of mathematical functions and avoids changing state and mutable data. However, you can use both paradigms together to create more robust software.
Working with Higher-Order Functions
Higher-order functions are a central concept in functional programming and a powerful tool in your coding arsenal. In a nutshell, a higher-order function is a function that can accept other functions as arguments or return a function as its result.
Example of Higher-Order Functions in Javascript
Here’s an example of a higher-order function used in Javascript:
Example Code | Description |
---|---|
function higherOrderFunction(callback) { | This function takes in another function (callback) as its argument and then calls it within its own code block. |
In this example, higherOrderFunction is a higher-order function. It accepts another function called callback as its argument and then calls it within its own code block.
Best Practices for Working with Higher-Order Functions
- Keep your higher-order functions simple and focused.
- Use anonymous functions or arrow functions for concise and readable code.
- Ensure that your higher-order functions return a new function rather than modifying the input function.
- Use higher-order functions to modularize your code and simplify complex logic.
By leveraging higher-order functions, you can write more flexible and reusable code, making it easier to scale and maintain your project.
Pure Functions and Immutability in Practice
In functional programming, pure functions and immutability are essential concepts that help write cleaner, more predictable code.
Pure Functions are functions that always produce the same output for a given input and do not modify any external state. In other words, they are stateless and have no side effects.
Here’s an example of a pure function:
Function | Input | Output |
---|---|---|
add | 2, 3 | 5 |
add | 4, 5 | 9 |
As you can see, the add function always returns the same output for a given input and has no side effects.
Immutability refers to the practice of not modifying data in place. Instead, you create new copies of the data when you need to make changes. This helps prevent unexpected changes to your data and makes your code easier to reason about.
Here’s an example of how to create an immutable object in Javascript:
const person = {
name: "John",
age: 30,
address: {
street: "123 Main St",
city: "New York",
state: "NY"
}
};
const updatedPerson = {
...person,
age: 31,
address: {
...person.address,
city: "Boston"
}
};
In this example, we create a new object updatedPerson based on the original person object, but with an updated age and a new address object. We do this using the spread operator (…) to copy all the properties of the original object and create a new one with the updated values.
By using pure functions and immutability, you can write more predictable, reliable code that is easier to maintain and debug.
Functional Data Transformation and Composition
Functional programming is all about transforming data from one form to another. In this section, we’ll explore different techniques for data transformation and function composition that can help you write cleaner and more maintainable code in Javascript.
Data Transformation
Data transformation is the process of converting data from one format to another. In functional programming, this is often achieved using higher-order functions such as map, reduce, and filter.
The map function is used to transform each element of an array using a provided function. For example:
const numbers = [1, 2, 3, 4];
const doubledNumbers = numbers.map(num => num * 2);
The above code will result in doubledNumbers
being equal to [2, 4, 6, 8]
.
The reduce function is used to reduce an array to a single value using a provided function. For example:
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((acc, num) => acc + num, 0);
The above code will result in sum
being equal to 10
.
The filter function is used to filter out elements of an array that don’t meet a specific condition. For example:
const numbers = [1, 2, 3, 4];
const evenNumbers = numbers.filter(num => num % 2 === 0);
The above code will result in evenNumbers
being equal to [2, 4]
.
Function Composition
Function composition is the process of combining multiple functions to create a new function. In functional programming, this is often achieved using higher-order functions such as compose and pipe.
The compose function is used to combine multiple functions from right to left. For example:
const add = x => x + 1;
const multiply = x => x * 2;
const addAndMultiply = compose(multiply, add);
const result = addAndMultiply(2);
The above code will result in result
being equal to 6
.
The pipe function is used to combine multiple functions from left to right. For example:
const add = x => x + 1;
const multiply = x => x * 2;
const addAndMultiply = pipe(add, multiply);
const result = addAndMultiply(2);
The above code will result in result
being equal to 6
.
Function | Use |
---|---|
map | Transform each element of an array using a provided function. |
reduce | Reduce an array to a single value using a provided function. |
filter | Filter out elements of an array that don’t meet a specific condition. |
compose | Combine multiple functions from right to left. |
pipe | Combine multiple functions from left to right. |
By mastering the techniques of data transformation and function composition, you can write cleaner and more maintainable code in Javascript. Keep exploring and applying these concepts to enhance your functional programming skills.
Error Handling in Functional Code
Errors are an inevitable part of software development. Proper error handling is critical for ensuring the reliability and robustness of your code. In functional programming, error handling is approached in a different way than in imperative programming.
In functional programming, errors are treated as values that can be propagated through a system using functional constructs. When an error occurs, it is handled by transforming the erroneous value into a result that represents the failure.
One way to handle errors in functional code is through the use of error monads, which are types that represent computations that can fail. By encapsulating the computation in a monad, error handling can be handled in a way that is transparent to the rest of the program.
Error Monads
Error monads provide a way to encapsulate computations that may fail. They allow you to handle errors in a way that is transparent to the rest of the program and provides a consistent means of error handling.
There are several common error monads, including the Maybe monad and the Result monad. The Maybe monad represents computations that may or may not produce a value, while the Result monad represents computations that may fail, producing an error value if they do.
When working with error monads, it’s important to handle errors in a way that provides feedback to the user. One way to do this is through the use of functional error handling libraries, such as folktale and ramda-fantasy. These libraries provide tools for handling errors in a way that is consistent with functional programming principles.
Handling Errors in Functional Code
When writing functional code, it’s important to handle errors in a way that is consistent with functional programming principles. This means treating errors as values that can be propagated through a system using functional constructs.
Some common strategies for handling errors in functional code include using error monads, wrapping functions with error-handling constructs, and using functional error handling libraries. By adopting these strategies, you can handle errors in a way that is transparent to the rest of the program and provides a consistent means of error handling.
Asynchronous Programming with Promises and Functional Style
Asynchronous programming is a necessary part of modern Javascript development. It allows us to perform time-consuming tasks, such as making API calls and loading large files, without blocking the user interface. Fortunately, Javascript provides us with Promises, a powerful language feature that simplifies asynchronous programming.
So, what exactly are Promises? In essence, Promises are placeholders for the results of asynchronous operations. They allow us to execute code when an operation finishes successfully or when it fails. Promises are chainable and can be composed into more complex operations, making them ideal for functional programming.
Here’s an example:
<script>
function fetchUser(userId) {
return fetch('https://api.example.com/users/' + userId)
.then(response => response.json())
.then(data => {
return {
firstName: data.firstName,
lastName: data.lastName,
email: data.email
};
});
}
fetchUser(123)
.then(user => {
console.log(user.firstName + ' ' + user.lastName);
})
.catch(error => {
console.error('Failed to fetch user', error);
});
</script>
In this example, we’re using Promises to fetch user data from an API. The fetchUser
function returns a Promise that resolves to an object containing the user’s first name, last name, and email. We can then use the then
method to execute code when the Promise resolves successfully.
Notice how the then
method returns a new Promise that resolves to a transformed version of the data. This is a crucial aspect of functional programming, as it allows us to create pure functions that don’t modify their input but instead return new values.
One problem with Promises is that they can become hard to manage when dealing with multiple asynchronous operations. This is where functional programming really shines. By breaking down complex operations into smaller, composable functions, we can write asynchronous code that is both easy to reason about and reusable.
Here’s an example of composing Promises into a more complex operation:
<script>
function fetchUsers(userIds) {
return Promise.all(userIds.map(fetchUser));
}
fetchUsers([123, 456, 789])
.then(users => {
// Display a list of users
})
.catch(error => {
console.error('Failed to fetch users', error);
});
</script>
In this example, we’re using the fetchUser
function from the previous example to fetch user data for multiple user IDs. The fetchUsers
function returns a Promise that resolves to an array of user data. We can then use the then
method to execute code when the Promise resolves successfully.
By combining small, composable functions that return Promises, we can write asynchronous code that is easy to reason about and maintain. This is the power of functional programming.
Testing and Debugging Functional Code
Testing and debugging are essential parts of the software development cycle. In functional programming, testing and debugging become even more critical because of the complexity of the code. In this section, we will explore techniques for testing and debugging functional code effectively.
Unit Testing
Unit testing is one of the most popular techniques for testing functional code. It involves testing individual units or functions of the code to ensure they are working as expected. Unit tests are automated and run every time a change is made to the codebase. This ensures that any issues are caught early and can be fixed quickly.
Functional code is generally easier to test than imperative code because it is more modular and has fewer side effects. However, testing still requires careful planning and attention to detail.
When writing unit tests for functional code, it is essential to test both the happy path and the edge cases. Edge case testing ensures that the code can handle unexpected inputs and edge cases.
Debugging Tools
Debugging functional code can be challenging because of its complexity. However, several tools can help make the process easier:
- console.log(): This is a simple yet effective debugging tool that prints messages to the browser console. It can help you understand how your code is behaving and identify issues.
- debugger statement: This is a powerful tool for debugging functional code. It can be used to pause the execution of code and inspect the state of variables and functions at a specific point in time.
- Chrome DevTools: DevTools is a comprehensive debugging tool that comes with Google Chrome. It can help you debug complex functional code and find issues quickly.
Functional Debugging Best Practices
Here are some best practices for debugging functional code:
- Use console.log() and debugger statements sparingly: Overusing these tools can make your code cluttered and challenging to understand.
- Write small, testable functions: Small functions are easier to test and debug than large ones.
- Refactor code: If you find yourself repeatedly using console.log(), it may be a sign that your code needs refactoring.
- Use property-based testing: Property-based testing involves testing your code against a set of properties rather than specific inputs and outputs. This can help you catch edge cases and other issues that are easy to miss with traditional testing.
Conclusion
In conclusion, mastering functional programming concepts with Javascript can bring immense benefits to your coding skills and web app development. As we learned throughout this guide, adopting a functional programming approach can lead to cleaner and more maintainable code, as well as simplifying complex tasks by utilizing concepts such as immutability, pure functions, higher-order functions, and recursion.
By embracing different functional programming paradigms, such as declarative programming, composition, and lazy evaluation, you can simplify your code further and make it more efficient. Working with higher-order functions, pure functions, and immutability can seem challenging at first, but with practice, it can become second nature and significantly improve the quality of your codebase.
Transforming data and composing functions are essential skills in functional programming, and effective error handling plays a crucial role in ensuring the reliability and correctness of your code. Asynchronous programming with promises in a functional style can make your code even more reliable and maintainable.
Finally, testing and debugging functional code is a must for any developer. With the strategies and tools we discussed, you can ensure that your code is bug-free and performing optimally. Keep exploring and applying these principles to further improve your Javascript development journey.
FAQ
What is functional programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It focuses on immutability, pure functions, higher-order functions, and recursion.
Why should I learn functional programming with Javascript?
Learning functional programming with Javascript can enhance your coding skills and make you a more efficient and effective developer. It allows you to write cleaner and more maintainable code, build dynamic web apps, and leverage the power of functional programming paradigms.
What are pure functions?
Pure functions are functions that always produce the same output for the same input and do not have any side effects. They rely only on their arguments and don’t modify anything outside their scope, making them predictable and easier to test.
What is immutability in functional programming?
Immutability refers to the concept of not changing data once it is created. In functional programming, immutable data is preferred because it simplifies code, improves performance, and reduces bugs caused by unintended modifications.
What are higher-order functions?
Higher-order functions are functions that can take other functions as arguments or return functions as results. They enable powerful abstractions and allow for more expressive and reusable code.
How can I handle errors in functional code?
Error handling in functional code can be done using approaches such as error monads and functional error handling libraries. These techniques allow you to handle errors in a functional and composable manner, ensuring the integrity of your code.
How can I test and debug functional code?
Testing and debugging functional code is essential for ensuring its correctness and reliability. You can use various strategies and tools specific to functional programming, such as property-based testing and functional debugging techniques.
Next tutorial: Understanding Fetch API and Web APIs in Javascript Mastery
Leave a Reply