Welcome to our comprehensive guide on how to use Knex with SQL. Whether you are new to development or a seasoned programmer, this guide will teach you everything you need to know about simplifying queries and optimizing performance.
Knex is a SQL query builder for Node.js, providing an intuitive way to interact with databases. SQL, on the other hand, is the language used to manage and manipulate relational databases. By using Knex with SQL, you can streamline your queries and make your application more efficient.
In this guide, we will take you through the process of setting up Knex and establishing a connection to your database. We will show you how to build queries using Knex’s fluent API, execute them, and optimize their performance. You will also learn advanced techniques for querying and manipulating data, as well as best practices for security and error handling.
With this guide, you will learn how to use Knex with SQL to its fullest potential, resulting in better performance and more efficient code. So let’s get started and see how easy it is to use Knex with SQL.
Introduction to Knex and SQL – Lean how to use Knex with sql
Welcome to our comprehensive guide on using Knex with SQL. Before we dive into the details, let’s first understand what Knex and SQL are, and how they work together to simplify database interactions.
What is Knex?
Knex is a query builder for Node.js that provides an intuitive and convenient way to interact with databases. It allows you to write SQL queries using JavaScript instead of raw strings, which makes the code easier to read, write, and maintain.
What is SQL?
SQL (Structured Query Language) is a language used to manage and manipulate relational databases. It is used to create, read, update, and delete data from tables, as well as perform complex operations such as filtering, sorting, and aggregating data.
Now that you have a basic understanding of Knex and SQL, let’s move on to the next section to learn how to set up Knex and establish a connection to your database.
Setting up Knex and Connecting to a Database
Before you can start using Knex with SQL, you need to get them both set up and connected. Follow these steps to ensure a smooth start to your project:
Step 1: Install Knex and a Database Driver
To get started with Knex, you’ll need to have it installed in your project. You can do this using npm. Open your terminal and run the following command:
npm install knex
You’ll also need a database driver. There are several drivers you can use, depending on your database of choice. Here are the most popular ones:
Database | Driver |
---|---|
PostgreSQL | pg |
MySQL | mysql |
SQLite | sqlite3 |
Microsoft SQL Server | mssql |
You can install the driver of your choice using npm. For example, if you’re using PostgreSQL, run the following command:
npm install pg
Step 2: Configure Knex
Once you have Knex and your database driver installed, you need to configure Knex to use the driver. Create a new file called knexfile.js in your project root directory and add the following code:
module.exports = {
client: 'pg',
connection: {
host: 'your_host_name',
user: 'your_username',
password: 'your_password',
database: 'your_database_name',
port: 'your_port_number'
}
};
Make sure you replace the placeholders with your database credentials.
Step 3: Establish a Connection
Now that Knex is configured, you can establish a connection to your database. In your project code, create a new instance of Knex by requiring it and passing in the configuration object:
const knex = require('knex');
const config = require('./knexfile.js');
const db = knex(config);
This creates a connection to your database that you can use to execute queries.
That’s it! You’re now ready to start creating and executing SQL queries with Knex.
Creating and Executing SQL Queries with Knex
Now that you have set up Knex and connected to your database, it’s time to start creating and executing SQL queries. Knex provides a fluent API for building SQL queries, making it easy to retrieve, insert, update, and delete data from your database.
To get started, let’s look at a simple example of retrieving data from a table using Knex:
Code:
const knex = require('knex')(config);
knex.select('*').from('users')
.where('name', 'John')
.then(rows => {
console.log(rows);
});
Description:
Selects all columns from the users table where the name is ‘John’, and logs the results to the console.
In the above example, we first initialize Knex with our database configuration object. We then use the select method to specify the columns we want to retrieve, and the from method to specify the table we want to query. The where method is used to add filters to our query, in this case filtering by the name column. Finally, we use the then method to execute the query and log the results to the console.
Inserting data is just as easy using Knex:
Code:
const knex = require('knex')(config);
knex('users').insert({name: 'John', email: 'john@example.com'})
Description:
Inserts a new row into the users table with the name ‘John’ and email ‘john@example.com’.
Updating and deleting data follow a similar pattern:
Code1:
const knex = require('knex')(config);
knex('users').where('id', 1).update({name: 'John Doe'})
Description:
Updates the name of the user with id 1 to ‘John Doe’.
Code2:
const knex = require('knex')(config); knex('users').where('id', 1).del()
Description:
Deletes the user with id 1 from the users table.
As you can see, building and executing SQL queries with Knex is straightforward and intuitive. With the help of Knex’s fluent API, you can simplify complex queries and maximize the performance of your application.
Query Building Techniques and Best Practices
Building efficient SQL queries takes more than just knowing the basics. To optimize your queries and improve the performance of your application, you need to apply the right techniques and follow industry best practices. Here are some of the key things to keep in mind when building your queries:
Use Prepared Statements
Prepared statements are a powerful tool for securing your SQL queries against common security threats such as SQL injection. They allow you to separate the SQL syntax from the user input, reducing the chances of malicious code being injected into your queries. Prepared statements are supported by Knex, so be sure to use them whenever possible.
Avoid SELECT *
It can be tempting to use SELECT * to retrieve all columns from a table, but this can have a significant impact on performance. Instead, explicitly list the columns you need in your query. This can help to reduce the amount of data that needs to be fetched from the database, resulting in faster query execution times.
Use Joins Sparingly
Joins can be a powerful tool for combining data from multiple tables, but they can also be a major performance bottleneck if used incorrectly. Avoid joining large tables whenever possible, and consider denormalizing your data to reduce the need for joins. If you do need to use a join, be sure to use the appropriate join type and create indexes on the columns being joined.
Limit the Results
Returning too many results can also have a negative impact on performance, especially if the result set is large. Use the LIMIT keyword to restrict the number of rows returned by your query, and consider using pagination to break up the results into smaller chunks.
Use Indexes
Indexes are a crucial tool for optimizing query performance. Be sure to create indexes on the columns that are frequently used in your queries, especially those involved in joins and WHERE clauses. Keep in mind that creating too many indexes can also have a negative impact on performance, so use them judiciously.
Avoid Subqueries
Subqueries can be a powerful tool for creating complex queries, but they can also be a major performance bottleneck if used incorrectly. Avoid using subqueries whenever possible, and consider using JOINs instead. If you do need to use a subquery, be sure to optimize it for performance by using appropriate indexes and limiting the result set.
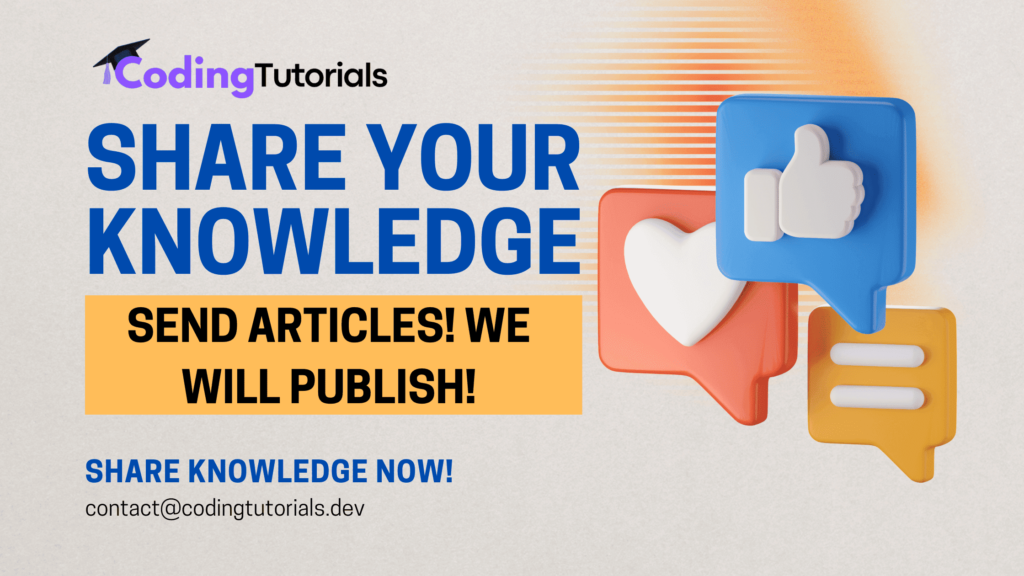
Migrations and Database Schema Management
Managing database schema changes is a crucial task in any application development. With Knex, you can automate this process using migrations. Migrations allow you to perform version control on your database schema, making it easy to update or roll back changes as needed.
To create a migration file, use the following command in your terminal:
npx knex migrate:make migration_name
Replace migration_name with a descriptive name for your migration. This command will create a new migration file in the migrations folder of your project.
Each migration file contains two functions: up and down . The up function defines the changes to be made to the database schema, while the down function is used to revert those changes.
Here is an example of a migration file that creates a new table called “users”:
File name: 20211213123456_create_users_table.js
Code:
exports.up = function(knex) {
return knex.schema.createTable('users', function(table) {
table.increments('id');
table.string('username').notNullable();
table.string('email').notNullable().unique();
table.string('password').notNullable();
table.timestamps(true, true);
});
};
exports.down = function(knex) {
return knex.schema.dropTable('users');
};
Once you have created your migration file, you can apply the changes to the database using the following command:
npx knex migrate:latest
This command will run all pending migrations and update the database schema accordingly. You can also roll back the last migration using the following command:
npx knex migrate:rollback
You can view the migration history and status using the following command:
npx knex migrate:status
With the help of migrations, you can keep your database schema in sync with your application’s evolving needs.
Working with Raw SQL and Transactions
Although Knex provides a convenient query builder, there may be situations where you need to execute raw SQL statements or work with transactions. Raw SQL statements allow you to execute SQL code directly on your database. Transactions allow you to group a set of SQL statements so that they either succeed or fail together.
Executing Raw SQL Statements with Knex
To execute raw SQL statements with Knex, you can use the knex.raw
method. This method allows you to execute any SQL code supported by your database. For example, the following code executes a simple SELECT statement:
// Example of raw SQL query
knex.raw('SELECT * FROM users').then(function(result) {
console.log(result);
});
You can also use the knex.schema.raw
method to execute raw SQL statements related to database schema modifications. For example, the following code creates a new table using a raw SQL statement:
// Example of raw SQL schema modification
knex.schema.raw('CREATE TABLE IF NOT EXISTS products (id INTEGER PRIMARY KEY, name TEXT)').then(function(result) {
console.log(result);
});
When using raw SQL statements, it’s important to keep in mind that you need to correctly escape any user input to prevent SQL injection attacks.
Implementing Transactions with Knex
To work with transactions in Knex, you can use the knex.transaction
method. The knex.transaction
method takes a callback function that receives a trx
object as its argument. You can then execute your SQL statements inside the callback function and use the trx
object to commit or rollback the transaction.
For example, the following code uses a transaction to insert a new user and a new product into the database. If either of the inserts fail, the entire transaction will be rolled back:
// Example of using transactions with Knex
knex.transaction(function(trx) {
return trx.insert({name: 'John'}).into('users')
.then(function(userIds) {
return trx.insert({name: 'Apple'}).into('products');
});
}).then(function() {
console.log('Transaction complete.');
}).catch(function(err) {
console.error(err);
});
Using transactions can help you ensure data consistency and reliability in your application.
Querying and Manipulating Data with Knex.js and SQL
As you become more familiar with Knex.js and SQL, you will need to learn advanced techniques for querying and manipulating data. In this section, we will explore some of these techniques, including joins, aggregations, subqueries, and advanced filtering options.
Joins
Joins allow you to combine data from two or more tables into a single result set. There are several types of joins, including inner join, left join, right join, and full outer join. Here’s an example of an inner join using Knex:
knex('users')
.join('orders', 'users.id', '=', 'orders.user_id')
.select('users.*', 'orders.order_date');
This query joins the users and orders tables on the users.id and orders.user_id columns and selects the user’s information and the order date. You can replace the ‘join’ method with ‘leftJoin’, ‘rightJoin’, or ‘fullOuterJoin’ to use a different type of join.
Aggregations
Aggregations allow you to perform calculations on groups of rows and return a summary result. Knex.js provides a variety of aggregation functions, including count, sum, avg, min, and max. Here’s an example of using the count function to count the number of orders each user has:
knex('users')
.join('orders', 'users.id', '=', 'orders.user_id')
.select('users.name', knex.raw('count(*) as order_count'))
.groupBy('users.id');
This query joins the users and orders tables on the users.id and orders.user_id columns, selects the user’s name, and counts the number of orders for each user. It then groups the results by the user’s id. You can replace the count function with any other aggregation function to calculate different summaries of your data.
Subqueries
Subqueries allow you to include the result of one query in another query. You can use subqueries to filter, join, or aggregate data. Here’s an example of using a subquery to filter orders by their total price:
knex('orders')
.whereIn('user_id', function() {
this.select('id').from('users').where('age', '>', 18);
})
.where('total_price', '>', 100);
This query selects orders where the user is over 18 years old and the total price is greater than 100. The subquery selects users with an age greater than 18 and returns their ids to the main query. You can use subqueries in a variety of ways to filter, join, or aggregate data.
Advanced Filtering
Knex.js provides a variety of methods for filtering data beyond basic where clauses. For example, you can use the whereBetween method to filter data between two values:
knex('orders')
.whereBetween('order_date', ['2021-01-01', '2021-12-31']);
This query selects orders where the order date is between January 1, 2021, and December 31, 2021. You can also use the whereNull and whereNotNull methods to filter data where a column is null or not null:
knex('users')
.whereNull('email');
This query selects users where the email column is null. You can also negate the condition using the whereNotNull method.
Securing Your SQL Queries with Knex
As with any application, security is a critical aspect when it comes to accessing your database through Knex.js and SQL. Common security vulnerabilities, such as SQL injection, can have a severe impact on your system’s security, which is why it’s crucial to take measures to protect your application.
Prepared Statements
One of the most effective ways to prevent SQL injection attacks is by using prepared statements. Prepared statements help prevent malicious SQL code from being executed by separating the SQL logic from the user input data.
Here’s an example of a prepared statement in Knex:
SQL Code | Knex.js Code |
---|---|
SELECT * FROM users WHERE name = ? | knex.select().from(‘users’).where(‘name’, ‘=’, req.body.name); |
In the above example, the user input is passed through the Knex.js API, which automatically handles the preparation and parameterization of the statement, preventing any malicious input from being executed.
Other Security Measures
In addition to using prepared statements, Knex.js also offers other security measures to protect your application, such as:
- Transaction management: Knex.js provides built-in support for transactions, which can help ensure data consistency and prevent partial commits.
- Input validation: Always validate user input before passing it to Knex.js to ensure that it meets the expected data format.
- Authentication and Authorization: Always implement proper user authentication and authorization to prevent unauthorized access to your system.
By taking these security measures, you can make your Knex.js and SQL queries more secure, preventing security vulnerabilities that can have a severe impact on your system’s security.
Performance Optimization with Knex and SQL
Optimizing the performance of your application is crucial for user satisfaction and overall success. In this section, we’ll explore some techniques for optimizing the performance of your SQL queries using Knex.
Query Caching
One way to improve performance is by implementing query caching. Query caching stores the results of a query in memory so that subsequent requests for the same query can be served faster.
To implement query caching with Knex, you can use a caching library like Redis or Memcached. These libraries can be used to store the query results and retrieve them quickly, reducing the load on your database and improving overall performance.
Connection Pooling
Another way to optimize performance is by using connection pooling. Connection pooling allows you to reuse database connections instead of creating a new connection for each request.
To use connection pooling with Knex, you can set the poolSize option in the configuration object. This option determines the maximum number of connections that can be open simultaneously.
Using Indexes Effectively
Indexes are an essential feature of any database, and they can significantly improve query performance. Properly using indexes can speed up data retrieval and reduce the load on your database.
When using Knex, you can create indexes by defining them in your migration files. It’s recommended to create indexes on columns that are frequently used for filtering or sorting data.
SQL Optimization
Optimizing your SQL queries is an essential part of improving performance. Here are some tips for optimizing your queries:
- Use EXPLAIN to analyze query performance and identify slow queries.
- Avoid using SELECT * as it can slow down data retrieval.
- Use WHERE clauses to filter data before it’s retrieved.
- Reduce the number of joins in your queries to improve performance.
Error Handling and Debugging with Knex and SQL
As much as we try to avoid them, errors are an inevitable part of software development. In this section, we will cover how to handle errors that may occur when working with Knex and SQL. We will also discuss debugging techniques that can help identify and resolve issues quickly.
Handling Errors with Knex
When using Knex, errors can occur for various reasons, such as incorrect syntax, invalid data types, or connection failures. To handle errors gracefully, we can use the try-catch block. The try block contains the code that may generate an error, and the catch block catches the error and performs appropriate actions, such as logging the error or displaying an error message to the user.
Here is an example of using the try-catch block in a Knex query:
try {
await knex('users')
.where('id', id)
.update({ name: 'John Doe' });
} catch (error) {
console.error(error);
}
This code attempts to update a user’s name in the database. If an error occurs, it is caught in the catch block, and the error is logged to the console.
Debugging SQL Queries
Debugging SQL queries can be challenging, especially when dealing with complex queries or large datasets. However, Knex provides several useful features for debugging, such as query logging and debugging helpers.
To enable query logging in Knex, we can use the debug option. When enabled, Knex logs all executed SQL queries to the console, making it easy to identify any errors or performance issues. We can also use the toSQL method to output the generated SQL statement for debugging purposes.
Here is an example of enabling query logging and using the toSQL method in a Knex query:
const query = knex.select('*').from('users').where('age', '>', 18).toSQL(); console.log(query);
This code generates a SQL query to select all users with an age greater than 18. The toSQL method outputs the generated SQL statement to the console.
In addition to query logging, Knex provides several debugging helpers that can simplify the debugging process. These helpers include debug, timeout, returning, and wrapIdentifier, among others.
Conclusion
Congratulations! You have now completed the comprehensive guide for using Knex with SQL. We hope you have gained valuable insights on how to simplify queries and maximize the performance of your application using Knex.
In this guide, you have learned the basics of Knex and SQL, how to set up Knex, and connect it to a database. You have learned how to create and execute SQL queries, build query techniques, and implement best practices. Moreover, you have discovered the importance of managing database schema changes and how to automate this process using migrations.
We have also covered working with raw SQL and transactions, querying, and manipulating data using Knex.js and SQL, securing SQL queries, and performance optimization techniques with Knex and SQL. In addition, you have gained insight into error handling and debugging with Knex and SQL.
With this knowledge, you are now equipped to use Knex with SQL, creating efficient and secure database queries and optimizing their performance. We wish you the best of luck on your journey to becoming a master in using Knex with SQL.
FAQ
What is Knex?
Knex is a SQL query builder for Node.js that provides a convenient and intuitive way to interact with databases.
What is SQL?
SQL (Structured Query Language) is a language used to manage and manipulate relational databases.
How do I set up Knex and connect to a database?
To set up Knex and establish a connection to your desired database, you need to configure the necessary settings. We will guide you through this process and show you how to test the connection.
How do I create and execute SQL queries with Knex?
Once you have Knex set up, you can start building SQL queries using Knex’s fluent API. We will teach you how to execute queries to retrieve, insert, update, and delete data from your database.
What are some query building techniques and best practices?
To optimize your SQL queries, it’s important to learn advanced techniques and follow best practices. We will cover topics such as query optimization, indexing, and avoiding common pitfalls that can impact your application’s performance.
How do I manage database schema changes with Knex?
Knex allows you to automate the process of managing database schema changes using migrations. We will explain how to create and run migrations to keep your database schema in sync with your application’s evolving needs.
Can I work with raw SQL and transactions with Knex?
Yes, Knex allows you to execute raw SQL statements and work with transactions when necessary. We will guide you through the process of using raw SQL and implementing transactional operations with Knex.
What advanced techniques can I use to query and manipulate data with Knex.js and SQL?
In this section, we will explore advanced techniques for querying and manipulating data using Knex.js and SQL. Topics covered include joins, aggregations, subqueries, and advanced filtering options.
How can I secure my SQL queries with Knex?
Security is important when dealing with SQL queries. We will discuss how to protect your application against common security vulnerabilities, such as SQL injection, by using prepared statements and other security measures provided by Knex.
How can I optimize performance with Knex and SQL?
To ensure optimal performance, it’s important to fine-tune your SQL queries and leverage Knex’s performance optimization features. We will explore techniques such as query caching, connection pooling, and using indexes effectively to enhance the speed and efficiency of your application.
How do I handle errors and debug with Knex and SQL?
Error handling and debugging are crucial skills in software development. We will teach you how to handle errors gracefully and troubleshoot common issues that may arise when working with Knex and SQL. We will also cover logging and debugging techniques to aid in the development and maintenance of your application. Happy coding!
Check our next tutorial: Javascript variables
3 Comments