Welcome to our comprehensive guide on implementing JWT access and refresh token authentication with Django and React. In today’s digital age, web app security is of utmost importance, and token-based authentication is one of the most secure methods of authentication available. With the combination of Django and React, we can achieve a powerful, secure, and scalable authentication system, thanks to the simplicity and flexibility of JSON Web Tokens (JWTs).
In this article, we will explore the concept of JWT access and refresh token authentication and learn how to set it up using Django and React. We will dive into the structure of JWT tokens, their role in authentication, and the step-by-step process of setting up Django and React for token-based authentication. We will also cover best practices and provide tips on how to handle token expiration and refreshing, protecting routes and resources, and securely storing and revoking tokens.
By the end of this article, you will have a deep understanding of JWT access and refresh token authentication and will be able to implement it in your own Django and React applications. So, let’s get started!
Keywords: JWT access and refresh token authentication, Django, React
JWT Access and Refresh Token Authentication in React: What are JWT Tokens?
JWT tokens or JSON Web Tokens are a type of token commonly used in web-based authentication processes. These tokens are encoded JSON objects that are signed with a secret key or public/private key pair, making them tamper-proof and verifiable.
JWT tokens consist of three parts: the header, payload, and signature. The header contains information about the type of token and the algorithm used for signature generation. The payload contains the user data and other information, while the signature is a hash of the header, payload, and the secret key or key pair.
One of the primary advantages of JWT tokens is their scalability and flexibility. They can be used to implement authentication across multiple systems and applications, making them a popular choice for modern web-based authentication processes.
Overall, JWT tokens are a powerful tool for enhancing the security of web applications. By understanding their structure and how they are used in authentication processes, developers can implement robust and secure authentication mechanisms to protect sensitive resources.
The Role of JWT Tokens in Authentication
Authentication is a crucial aspect of web application security. The process of authenticating users and authorizing access to protected resources involves the use of various techniques and protocols. One such technique is JWT token authentication, which uses JSON Web Tokens as a means of authentication.
JWT tokens play a significant role in this process by storing user information in a secure manner and providing access to protected resources. They are used for both access and refresh token authentication, and are structured using three distinct parts: header, payload, and signature.
Access Tokens
Access tokens are used to authenticate users and gain access to protected resources. Once a user logs in, a token containing user-specific information is generated and returned to the user. This token is then included in subsequent requests to protected resources to prove that the user is authorized to access them.
One of the advantages of using access tokens is that they can be easily revoked or invalidated if necessary. This can be done by maintaining a blacklist of tokens that have been revoked.
Refresh Tokens
Refresh tokens are used to obtain new access tokens without requiring the user to authenticate again. They are typically long-lived tokens that are issued alongside access tokens and can be used to request a new access token when the old one expires.
Refresh tokens are particularly useful in scenarios where access tokens have a short lifespan or are issued for a limited period of time. By using a refresh token, the user can continue to access protected resources without having to log in again.
In summary, JWT tokens play a vital role in enhancing web app security by providing a secure means of authentication and authorization. By understanding the role of JWT tokens in authentication and implementing them correctly, you can ensure that your web app is secure and protected from unauthorized access.
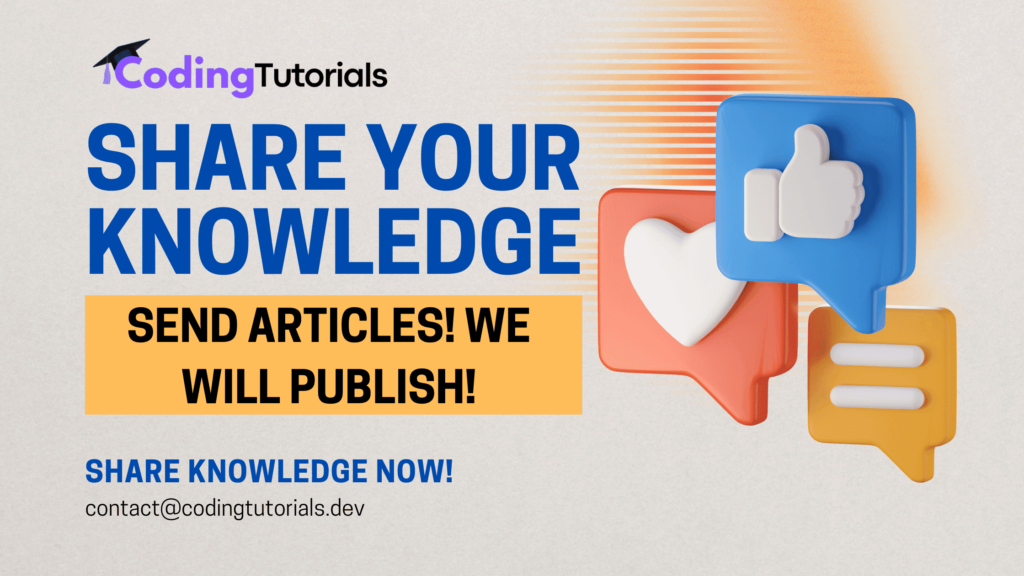
Setting up Django for JWT Token Authentication
Implementing JWT token authentication in Django requires a few essential setup steps. Here, we will guide you through the process, ensuring that you have all the necessary tools to secure your web app.
Step 1: Installation of Necessary Packages
First, install the following packages using pip:
- djangorestframework_simplejwt
- PyJWT
These packages are essential for enabling JWT token authentication in Django.
Step 2: Configuration in the Django Settings File
After installation, open the settings.py file of your Django project. Add the following code:
Code Snippet | Description |
---|---|
SIMPLE_JWT = { ‘ACCESS_TOKEN_LIFETIME’: datetime.timedelta(minutes=15), ‘REFRESH_TOKEN_LIFETIME’: datetime.timedelta(days=1), ‘ROTATE_REFRESH_TOKENS’: True, ‘BLACKLIST_AFTER_ROTATION’: False, ‘ALGORITHM’: ‘HS256’, ‘SIGNING_KEY’: settings.SECRET_KEY, ‘VERIFYING_KEY’: None, ‘AUTH_HEADER_TYPES’: (‘Bearer’,), ‘USER_ID_FIELD’: ‘id’, ‘USER_ID_CLAIM’: ‘user_id’, ‘AUTH_TOKEN_CLASSES’: (‘rest_framework_simplejwt.tokens.AccessToken’,), ‘TOKEN_TYPE_CLAIM’: ‘token_type’, } | This code snippet configures the Simple JWT library’s settings, setting token lifetime, token rotation, algorithm, signing, and other essential properties. |
Step 3: Create Views and Endpoints for Token Generation
Create views and endpoints for token generation by following these steps:
- Create a new file called views.py
- Add the following code:
Code Snippet | Description |
---|---|
from rest_framework_simplejwt.views import TokenObtainPairView, TokenRefreshView
class MyTokenObtainPairView(TokenObtainPairView): class MyTokenRefreshView(TokenRefreshView): | This code imports simple JWT’s token obtain and refresh views. It also defines custom views for token generation and refreshing, setting custom serializer classes. |
- Create a new file called serializers.py
- Add the following code:
Code Snippet | Description |
---|---|
from rest_framework_simplejwt.serializers import TokenObtainPairSerializer, TokenRefreshSerializer
class MyTokenObtainPairSerializer(TokenObtainPairSerializer): class MyTokenRefreshSerializer(TokenRefreshSerializer): | This code imports simple JWT’s token obtain and refresh serializers. It also creates custom serializer classes that inherit the existing serializers. |
- Add the following lines to your urls.py file:
Code Snippet | Description |
---|---|
from django.urls import path from .views import MyTokenObtainPairView, MyTokenRefreshView urlpatterns = [ | This code creates endpoints for token obtainment and refresh. It maps the custom views we created earlier to these URLs. |
With these steps, you should now be able to generate access and refresh JWT tokens in Django.
Implementing JWT Token Authentication in React
Now that we have set up JWT token authentication in Django, the next step is to implement it in our React app. This will allow us to send and receive tokens from the backend, store them securely, and handle token expiration and renewal.
We’ll start by creating a new file in our React app called auth.js. This file will contain all the functions we need to handle authentication, including logging in, logging out, and checking if a user is authenticated.
First, we’ll create a function called login() that will make a POST request to our Django backend to retrieve a JWT access token. We can then store this token in local storage or a cookie to keep the user authenticated.
Here’s an example of what our login() function might look like:
async function login(username, password) {
try {
const response = await fetch('/api/token/', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
username: username,
password: password
})
});
if (!response.ok) {
throw new Error('Invalid credentials');
}
const data = await response.json();
localStorage.setItem('access_token', data.access);
} catch (error) {
console.error('Login failed:', error.message);
}
}
In this example, we make a POST request to our Django backend’s /api/token/ endpoint with the user’s credentials. We then store the access token in local storage using the localStorage.setItem() function.
Next, we’ll create a function called isLoggedIn() that will check if a user is authenticated by verifying the presence and validity of their access token. Here’s what this function might look like:
function isLoggedIn() {
const access_token = localStorage.getItem('access_token');
if (!access_token) {
return false;
}
try {
const decoded_token = jwt_decode(access_token);
const current_time = Date.now() / 1000;
if (decoded_token.exp < current_time) {
localStorage.removeItem('access_token');
return false;
}
return true;
} catch (error) {
console.error('Error decoding token:', error.message);
return false;
}
}
In this example, we first retrieve the access token from local storage using the localStorage.getItem() function. If the token is not present, we return false. If the token is present, we decode it using the jwt_decode() function and check if it has expired by comparing the expiration time to the current time. If the token has expired, we remove it from local storage and return false. If the token is still valid, we return true.
Finally, we’ll create a function called logout() that will remove the user’s access token from local storage, effectively logging them out. Here’s what this function might look like:
function logout() {
localStorage.removeItem('access_token');
}
With these functions in place, we can now implement JWT token authentication throughout our React app. We can check if a user is authenticated before allowing them to access protected routes or resources, and we can handle token expiration and renewal by automatically requesting a new access token using their refresh token.
Protecting Routes and Resources with JWT Tokens
One of the significant advantages of using JWT tokens in authentication is the ability to protect routes and resources within your Django and React app. To achieve this, you need to verify the token’s authenticity and ensure that it has not expired.
To verify the token, your backend should decode the token using the same secret key used to sign it. If the signature is valid, you can grant access to protected routes and resources.
Creating middleware or higher-order components to check for authentication is a common practice in React. For instance, you can create a PrivateRoute component that takes in a component as a prop and checks if the user is authenticated. If the user is authenticated, it renders the component. Otherwise, it redirects the user to the login page.
Here’s an example:
<Route
{...rest}
render={({ location }) =>
isAuthenticated ? (
<Component />
) : (
<Redirect
to={{
pathname: "/login",
state: { from: location }
}}
/>
)
}
/>
Another useful technique is to handle unauthorized access scenarios gracefully. For instance, you can display a message informing the user that they are not authorized to access the resource and redirect them to a safe location on your app.
Table: Example of Protected Routes
Route | Protected |
---|---|
/dashboard | Yes |
/profile | Yes |
/login | No |
/signup | No |
In summary, protecting routes and resources with JWT tokens is a crucial step in enhancing the security of your Django and React app. By verifying the token’s authenticity, creating middleware or higher-order components, and handling unauthorized access scenarios, you can protect sensitive information and keep your app secure.
Handling Token Expiration and Refreshing Tokens
In JWT access and refresh token authentication, access tokens have a limited lifespan, after which they expire and become invalid. This is where refresh tokens come into play, allowing users to obtain new access tokens without re-authenticating. In this section, we will explore how to handle token expiration and implement token refreshing in your Django and React app.
Handling Token Expiration
When an access token expires, the user must obtain a new one to access protected resources. In Django and React, this can be handled by checking the token expiry time and issuing a new token if it has expired. To achieve this, we can create a middleware/hoc component to check the token’s expiration time before every request and renew the token if necessary.
Here’s some sample code:
<script type="text/babel">
class TokenMiddleware extends React.Component {
componentDidMount() {
this.checkTokenExpire();
}
checkTokenExpire = () => {
const token = window.localStorage.getItem('token');
const decodedToken = jwt.decode(token);
if (decodedToken.exp < Date.now() / 1000) {
this.refreshToken();
}
}
refreshToken = () => {
// code to request a new token and update local storage
}
render() {
return this.props.children;
}
}
export default TokenMiddleware;
Refreshing Tokens
Refresh tokens can be used to obtain new access tokens without the user re-authenticating. When an access token expires, the user sends a request with the expired token and the refresh token to the server. The server verifies the refresh token and issues a new access token.
To implement token refreshing in Django and React, we can create a function that sends a request to the server to refresh the token and updates the local storage with the new token. Here’s some sample code:
<script type="text/babel">
const refreshAccessToken = async () => {
const refreshToken = window.localStorage.getItem('refreshToken');
const response = await fetch('/api/token/refresh/', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({refresh: refreshToken})
});
const data = await response.json();
if (data.access) {
window.localStorage.setItem('token', data.access);
}
};
export default refreshAccessToken;
By handling token expiration and refreshing, we can ensure that our web app remains secure and accessible to authorized users. It’s important to follow best practices for token expiration policies and secure token storage to prevent unauthorized access.
Best Practices for JWT Access and Refresh Token Authentication
Implementing JWT access and refresh token authentication is an effective way to enhance the security of your web app. However, to ensure that your authentication system is as secure as possible, it’s important to follow the best practices outlined below.
1. Set Token Expiration Policies
Setting token expiration policies helps to prevent unauthorized access to resources by ensuring that tokens are invalidated after a certain period of time. It’s recommended that access tokens have a short expiration time, typically between 5 and 30 minutes, while refresh tokens have a longer expiration time. This helps to limit the window of opportunity for a potential attacker to use a stolen token.
2. Store Tokens Securely
Storing tokens securely is crucial to prevent them from being compromised. Access tokens should be stored in a secure HTTP-only cookie or in local storage, while refresh tokens should be stored in an HTTP-only cookie with the secure attribute set to true. This helps to prevent cross-site scripting (XSS) and cross-site request forgery (CSRF) attacks.
3. Implement Token Revocation
Implementing token revocation allows you to manually invalidate tokens if they are compromised or if a user logs out. It’s recommended that you keep track of the tokens issued and revoke any tokens that are no longer needed. This helps to limit the damage that can be caused by a stolen token.
4. Update Cryptographic Keys Regularly
Updating cryptographic keys regularly helps to enhance security by preventing or limiting the damage of a cryptographic attack. It’s recommended that you use a key rotation policy to update keys at regular intervals, such as every month or every quarter. This ensures that your system is protected against attacks that exploit weaknesses in cryptographic algorithms.
5. Use HTTPS
Using HTTPS helps to protect your web app from man-in-the-middle (MITM) attacks and ensures that all data transmitted between the client and server is encrypted. It’s recommended that you use HTTPS for all communication between the client and server, not just for the authentication process. This helps to prevent attackers from intercepting or tampering with sensitive data.
6. Use JSON Web Encryption (JWE) for Sensitive Data
If your web app handles sensitive data, it’s recommended that you use JSON Web Encryption (JWE) to encrypt the data before transmitting it. JWE is a method of encrypting JSON data that provides confidentiality, data integrity, and authenticity. This helps to prevent sensitive data from being intercepted or tampered with.
By following these best practices, you can ensure that your JWT access and refresh token authentication system is as secure as possible. Always keep security in mind when designing and implementing your authentication system.
Conclusion
Now that you have learned how to implement JWT access and refresh token authentication using Django and React, it’s time to take action and secure your web app. By following the step-by-step guide provided in this article, you can ensure the protection of sensitive resources and prevent unauthorized access.
Remember to adhere to best practices such as implementing token expiration policies, secure token storage, and regular updates of cryptographic keys. These measures will help to enhance the security of your authentication system.
Thank you for taking the time to read this article. We hope that you have found it informative and useful. If you have any questions or comments, please feel free to reach out to us. We wish you the best of luck in implementing JWT token authentication!
FAQ
What are JWT tokens?
JWT tokens are JSON Web Tokens that are used in authentication processes. They consist of three parts – the header, the payload, and the signature. JWT tokens are commonly used for securing web applications and APIs.
What is the role of JWT tokens in authentication?
JWT tokens play a crucial role in authentication. Access tokens are used to authenticate users and authorize access to protected resources, while refresh tokens aid in obtaining new access tokens without requiring the user to authenticate again.
How do I set up Django for JWT token authentication?
To set up Django for JWT token authentication, you need to install the necessary packages, configure your Django settings file, and create views and endpoints for token generation. This allows Django to issue and verify JWT tokens for authentication purposes.
How can I implement JWT token authentication in a React application?
Implementing JWT token authentication in a React application involves sending and receiving tokens from the backend, securely storing them in local storage or cookies, and handling token expiration and renewal. This ensures that authenticated users can access protected resources.
How can JWT tokens be used to protect routes and resources?
JWT tokens can be used to protect routes and resources by verifying the authenticity and validity of the tokens. This can be accomplished by creating middleware or higher-order components to check authentication and handle unauthorized access.
How do I handle token expiration and refresh tokens?
To handle token expiration, you can use refresh tokens to obtain new access tokens. This involves implementing token refreshing mechanisms in both Django and React to ensure uninterrupted access to protected resources.
What are some best practices for JWT token authentication?
Some best practices for JWT token authentication include setting token expiration policies, securely storing tokens, implementing token revocation mechanisms, and regularly updating cryptographic keys. It is essential to follow these practices to ensure the security of your authentication system.
Happy coding!
Next Tutorial: Exploring Context API in React Js: With easy steps
Leave a Reply