Welcome to the ultimate guide to mastering Progressive Web Apps (PWAs) and Service Workers in Javascript! In today’s fast-paced world, creating web applications that are both performant and engaging has become more critical than ever. PWAs and Service Workers are two powerful technologies that can revolutionize the way you develop web applications, and this guide will teach you everything you need to know to get started.
First, we will explore the concept of Progressive Web Apps and Service Workers, and how they can benefit your web development skills. We will then dive into the practical aspects of building PWAs and explore how Service Workers can take them to the next level. Finally, we will address important topics such as performance optimization, security considerations, testing, deploying, and the future prospects of PWAs and Service Workers in Javascript.
Whether you are a seasoned developer or just starting with web development, this guide is an invaluable resource that will help you enhance your knowledge and skills in building progressive web applications. So let’s get started and master Progressive Web Apps (PWAs) and Service Workers in Javascript!
What are Progressive Web Apps?
Progressive Web Apps (PWAs) are a new approach to building web applications that deliver a highly engaging and native-like experience to users. Unlike traditional web apps, PWAs can work offline, load instantly, and provide seamless navigation, resulting in a superior user experience.
Key Features of PWAs
- Progressive: Built with progressive enhancement as a core principle, PWAs can work on any device, regardless of the browser or operating system.
- Responsive: PWAs can adapt to any screen size, ensuring a consistent and visually appealing layout on all devices.
- App-like: PWAs behave like native apps, allowing users to add them to their home screen, receive push notifications, and even work offline.
- Discoverable: PWAs are easy to find and share, as they can be indexed by search engines and shared via URL.
- Secure: PWAs are served over HTTPS, ensuring a secure and trustworthy browsing experience for users.
Benefits of PWAs for Users and Developers
PWAs offer many benefits to both users and developers. Here are some of the most significant advantages:
Users | Developers |
---|---|
Fast loading times, even on slow networks | Reduced development costs and time-to-market |
Seamless offline functionality | Improved user engagement and retention |
Native-like experience | Easy maintenance and updates |
Ability to add to home screen | Greater visibility and discoverability |
Push notifications | Access to new APIs and functionalities |
With these benefits, it’s no wonder why PWAs are quickly gaining popularity among businesses and developers alike. In the next section, we’ll explore the fundamentals of Service Workers, an essential component of PWAs.
Introduction to Service Workers in javascript
Service Workers in javascript are a powerful JavaScript API that enables various functionalities for Progressive Web Apps (PWAs). They act as a proxy between the browser and the network, intercepting network requests and enabling offline caching, background synchronization, and push notifications.
Fun Fact: Service Workers were first introduced in 2014 as part of the Web Workers API and have since become an integral part of modern web development.
Capabilities of Service Workers
Service Workers have several capabilities that make them key to the success of PWAs. They can:
- Cache important resources for offline use, including HTML, CSS, and JavaScript files
- Intercept network requests and provide custom responses based on cache availability
- Enable push notifications for real-time updates and engagement
- Synchronize data in the background, even when the app is not actively in use
- Improve overall performance and reliability of PWAs
Offline Caching with Service Workers
One of the most significant benefits of Service Workers is their ability to provide offline caching for PWAs. By caching critical resources, Service Workers ensure that users can continue to access and use the app even when they’re offline or experiencing network connectivity issues.
A common approach to caching with Service Workers is to use the Cache API to create a cache of static assets such as HTML, CSS, and JavaScript files. This approach allows the app to load quickly and provide a seamless user experience, even in low connectivity situations.
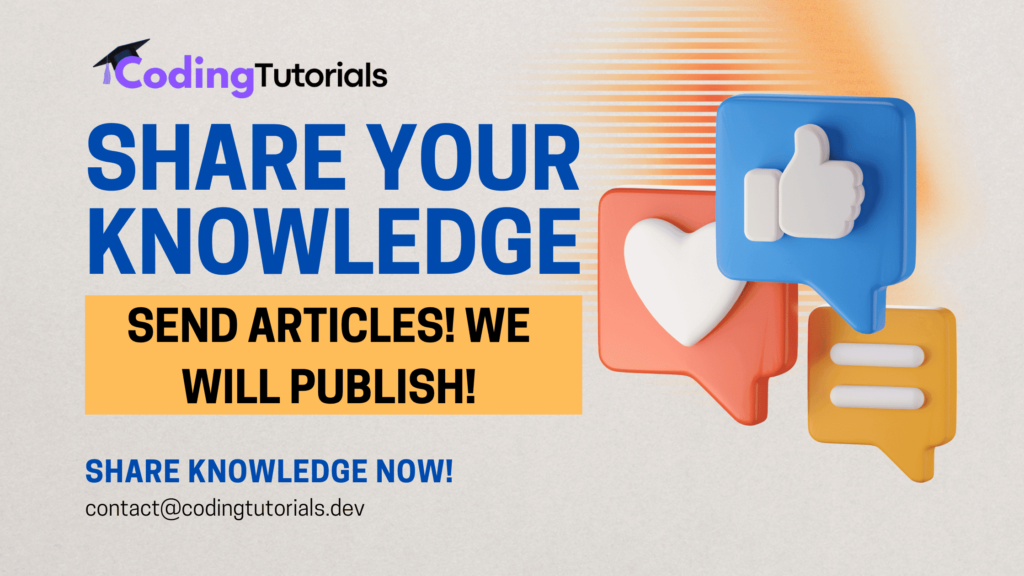
Background Synchronization with Service Workers
Another powerful capability of Service Workers is background synchronization. This feature allows PWAs to synchronize data with remote servers in the background, even when the app is not actively in use.
Background synchronization ensures that the app remains up-to-date with the latest data and enables real-time collaboration and engagement. It also reduces the need for constant user interaction and provides a smoother and more efficient user experience.
Push Notifications with Service Workers
Push notifications are an essential feature of modern web apps, and Service Workers make it easy to implement them in PWAs. Push notifications enable real-time updates and engagement with users, even when they’re not actively using the app.
Service Workers can intercept push notifications and customize how they’re displayed to users. This allows you to provide a personalized and engaging experience for your users, increasing their loyalty and satisfaction.
Getting Started with PWAs
Now that we understand the concept of Progressive Web Apps (PWAs), it’s time to dive into the practical aspects of building them. In this section, we will explore the fundamental requirements for creating PWAs and the best practices for developing them.
Web App Manifests
A web app manifest is a JSON file that defines metadata for your PWA, such as the app name, icon, and theme color. It also enables features like “Add to Home Screen,” which allows users to launch your PWA from their device’s home screen.
Here’s an example of a sample manifest:
Property | Description |
---|---|
name | The name of the app |
short_name | A shorter name for the app (optional) |
start_url | The URL to load when the app is launched |
icons | An array of image objects representing the app’s icon |
theme_color | The color of the app’s UI |
Service Worker Registration
A Service Worker is a type of Web Worker that runs separately from the main browser thread, enabling offline caching, push notifications, and background synchronization. To register a Service Worker, you need to add the following code to your app:
- Create a JavaScript file for your Service Worker
- Register the Service Worker in your app’s JavaScript file
- Add event listeners for the Service Worker’s lifecycle events
Here’s an example of how to register a Service Worker:
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js')
.then(registration => {
console.log('Service Worker registered!');
})
catch(error => {
console.error('Registration failed:', error);
});
});
}
Responsive Design
One of the key benefits of PWAs is that they are designed to work on any device and any screen size. To achieve this, you need to create a responsive design that adapts to different viewport sizes. This includes using relative units like percentages and ems, setting breakpoints for different screen sizes, and using CSS media queries to apply different styles based on the device’s characteristics.
By following these best practices for building PWAs, you can create engaging, offline-ready web applications that provide a seamless user experience.
Enhancing PWAs with Service Workers
Progressive Web Apps (PWAs) offer a rich set of features to create immersive and engaging web experiences. One of the key components of PWAs is Service Workers, a powerful JavaScript API that enables various functionalities for offline caching, background synchronization, and push notifications. By utilizing Service Workers, you can enhance the performance and reliability of your PWAs, providing seamless user experiences regardless of network conditions.
Offline Support
Service Workers allow you to cache critical resources of your PWA, such as HTML, CSS, and JavaScript files, making them available even when the user is offline. This feature enables your PWA to load instantly, without requiring a network connection. If you’re building a PWA that requires consistent access to data, caching can significantly improve the user experience, reducing loading times and increasing engagement.
Background Synchronization
Service Workers allow you to schedule background tasks that can synchronize data between your PWA and a server. This feature is particularly useful for PWAs that rely on real-time data, such as chat applications, social media platforms, and calendars. By using background synchronization, your PWA can remain up-to-date even when the user is not actively using it.
Push Notifications
Service Workers enable push notifications, allowing your PWA to send timely and relevant messages to users, even when the PWA is not open in a browser window. Push notifications are a powerful way to re-engage users and increase retention rates. Your PWA can utilize push notifications to notify users of relevant updates, such as new messages, new content, or important events.
Effective Caching
Service Workers require careful management of the cache to ensure that the data stored is up-to-date and relevant. Utilizing the Cache API, you can customize how your Service Worker caches data, specifying which resources to cache and when to update them. Effective caching can significantly improve the performance of your PWA, reducing loading times and network usage.
By leveraging the power of Service Workers, you can take your PWAs to the next level, enhancing their capabilities, reliability, and performance.
Optimizing Performance in PWAs
For PWAs to provide a seamless experience to users, it is crucial to optimize their performance. Slow loading speed and high network usage can deter users and reduce engagement. In this section, we will explore the best practices for performance optimization in PWAs.
Improving Loading Speed
One of the essential aspects of performance optimization is improving loading speed. Users expect fast-loading websites, and research shows that even a one-second delay in loading time can decrease conversion rates by up to 7%. Here are some techniques for improving loading speed:
- Minimize the size of your resources, such as images, scripts, and stylesheets.
- Use lazy loading for images and JavaScript files that are not critical for initial rendering.
- Optimize your code by removing unnecessary code, reducing the use of libraries, and avoiding synchronous network requests.
- Use a content delivery network (CDN) to serve your resources from a server closer to the user.
Reducing Network Usage
PWAs rely on network requests to fetch resources and data. However, excessive network usage can increase loading time, data costs, and user frustration. Here are some techniques for reducing network usage:
- Implement caching to store resources in the browser and avoid unnecessary network requests. Service Workers can enable effective caching by intercepting network requests and serving cached responses.
- Use data compression techniques, such as gzip, to reduce the size of data transferred over the network.
- Optimize the use of JavaScript to reduce the amount of data that needs to be transferred between the server and the browser.
Optimizing Rendering
Rendering is the process of transforming HTML, CSS, and JavaScript into a visible layout on the screen. Slow rendering can cause a delay in the visual display of the web page, leading to a poor user experience. Here are some techniques for optimizing rendering:
- Avoid using too many DOM elements, as this can slow down the rendering time.
- Implement responsive design to ensure that your web page renders correctly on different screen sizes and devices.
- Use CSS animations and transitions instead of JavaScript for basic animations, as they are faster and smoother.
By implementing these techniques, you can optimize the performance of your PWAs and provide a fast, responsive, and engaging experience to your users.
Security Considerations for PWAs
When building Progressive Web Apps (PWAs), it is important to consider the security implications of your design choices. Unlike traditional web apps, PWAs can be installed on a user’s device and may have access to sensitive information such as device data and user location. This section will cover some of the key security considerations for PWAs.
Secure Service Workers
Service Workers play a critical role in the functionality of PWAs, but they can also be a potential security risk. Malicious actors could inject malicious code into your Service Worker, compromising the security and privacy of your users. To mitigate this risk, it is important to ensure that your Service Workers are secure and properly validated.
When registering a Service Worker, make sure to use HTTPS to encrypt the connection and prevent network sniffing. Additionally, consider implementing code signing or other techniques to verify the integrity of your Service Worker code before deployment.
Handling Offline Data
Another security consideration for PWAs is how to handle offline data. When a user goes offline, data may be cached on their device for later use. This data can include sensitive information such as user credentials and payment details. Therefore, it is important to ensure that this data is securely stored and encrypted to prevent unauthorized access.
Consider implementing encryption techniques such as AES to protect the cached data. Additionally, be sure to include expiration dates in your cache to ensure that sensitive data is not stored for longer than necessary.
User Privacy
As with any web application, user privacy is a critical concern for PWAs. When requesting user permissions, such as device data or location access, be transparent about why you are collecting this information and ensure that it is necessary for the core functionality of your app.
Additionally, be sure to follow best practices for data protection, such as hashing or salting user passwords and securely transmitting data over the network.
Summary
In conclusion, building secure PWAs requires careful consideration of the potential security risks and implementation of best practices to mitigate them. By ensuring the security and privacy of your users, you can create PWAs that provide both engaging user experiences and peace of mind.
Testing and Debugging PWAs
Testing and debugging are crucial aspects of building PWAs. It ensures that your app works flawlessly across different devices and browsers. In this section, we will discuss some essential tools and techniques for testing and debugging PWAs.
Tools for testing PWAs
There are various tools available for testing PWAs across multiple devices and browsers. Here are some popular ones:
Tool | Description |
---|---|
Lighthouse | It is an open-source tool available in Chrome DevTools that audits your PWA for performance, accessibility, and other best practices. |
BrowserStack | It is a cloud-based testing platform that allows you to test your PWA on real devices and browsers. |
Selenium | It is an open-source framework for automating browser testing. You can use it to test your PWA across different browsers and platforms using different programming languages. |
Debugging Service Workers
Debugging Service Workers can be challenging as they run in a separate thread from the main browser context. However, the Chrome DevTools provide some useful features for debugging Service Workers. Here are some ways to debug Service Workers:
- Service Worker pane: Chrome DevTools provide a separate pane for Service Workers that allows you to inspect the cache, push subscriptions, and event listeners.
- Console logging: You can add console logging statements in your Service Worker code to debug specific parts of your code.
- Remote debugging: You can use the remote debugging feature in Chrome to connect to your Service Worker running on a remote device or browser.
By using these tools and techniques, you can ensure that your PWA is fully functional and delivers the best user experience.
Deploying and Publishing PWAs
After developing your Progressive Web App (PWA), the next step is to deploy and publish it to make it accessible to users. Deploying involves preparing your PWA for production, while publishing involves distributing it through various channels.
Deploying Your PWA
The first step in deploying your PWA is to optimize it for production. This involves performing checks for performance, security, and accessibility. Use tools such as Lighthouse, Google’s PWA auditing tool, to identify any issues and address them accordingly.
Once you have optimized your PWA, you can deploy it to your server. Ensure that your server is configured to serve the PWA’s resources from the correct location, and that the service worker is registered correctly.
To enable offline access, you also need to configure your server to respond with a fallback page when a user attempts to access a missing resource. This is referred to as a “404 fallback” and ensures that users can still access your PWA when they are offline.
Lastly, ensure that you have set up SSL/TLS encryption for your server to ensure data security, as this is a requirement for PWAs to be installed on a user’s device.
Publishing Your PWA
There are various channels through which you can publish your PWA. Here are some popular options:
- Your website: You can simply provide a link to your PWA on your website. This is the easiest method, but it requires users to visit your website to access the PWA.
- App store listings: You can package your PWA as a standalone app and submit it to app stores such as Google Play and the Apple App Store. This allows users to install your PWA like a native app, which can improve discoverability and user engagement.
- Chrome Web Store: You can also publish your PWA on the Chrome Web Store, which is a platform for web-based apps and extensions that can be used with the Google Chrome browser. This can help improve visibility and credibility for your PWA.
When publishing your PWA, ensure that you provide all the necessary information, such as app icons, descriptions, and screenshots, to entice users to install it. You can also optimize your PWA for search engines by including relevant keywords in your app title and description.
By deploying and publishing your PWA, you can make it available to a wider audience and take advantage of the benefits of PWAs, such as offline access and improved user engagement.
Advancements in PWAs and Service Workers
As the web technology landscape continues to evolve, so do the capabilities and potential of Progressive Web Apps and Service Workers. Let’s take a look at some of the latest advancements and emerging trends in this area.
WebAssembly
WebAssembly is a binary format for executing code on the web, providing a high level of performance that is ideal for computationally intensive tasks. WebAssembly modules can now be used within Service Workers, enabling web developers to create highly performant and responsive PWAs even for resource-intensive use cases such as gaming and video editing.
Push API Upgrades
The Push API has been updated to include features such as payload encryption, message bundling, and expiration timestamps. These enhancements improve security, reliability, and the overall user experience of push notifications.
Web Share Target API
The Web Share Target API enables users to share content from PWAs to native mobile apps, making it easier to integrate PWAs with the wider app ecosystem. This API allows PWAs to register themselves as share targets and handle incoming share requests from other apps.
Background Fetch API
The Background Fetch API allows PWAs to schedule periodic network requests and downloads even when the app is not actively running. This feature is particularly useful for apps that require frequent data updates, such as news or weather apps.
Web Payments
The Web Payments API enables PWAs to offer seamless payment experiences to users, integrating with a variety of payment providers and methods. This feature allows PWAs to offer a more seamless and integrated shopping experience, without requiring users to navigate outside the application.
Browser Support
Browser support for PWAs and Service Workers continues to grow, making it easier for developers to reach users across a wide range of devices and platforms. Popular browsers such as Chrome, Firefox, Safari, and Edge now fully support PWAs and Service Workers, enabling a consistent and reliable user experience.
Conclusion
Congratulations on completing this comprehensive guide to mastering Progressive Web Apps and Service Workers in Javascript.
By now, you should have a solid understanding of the core concepts, features, and best practices of building PWAs and working with Service Workers. You have learned how to create engaging and performant web applications that work seamlessly across various devices and networks.
Remember that the world of web development is constantly evolving, and there are always new tools and techniques to explore. Stay curious, keep learning, and experiment with different approaches to find what works best for you and your projects.
Keep Pushing the Boundaries of Web Development
As a web developer, you have the power to shape the digital experiences of millions of users around the world. By leveraging the potential of PWAs and Service Workers, you can create innovative and impactful web applications that are a joy to use.
Embrace new technologies, experiment with new ideas, and keep pushing the boundaries of web development. The possibilities are endless!
FAQ
What are Progressive Web Apps?
Progressive Web Apps (PWAs) are web applications that combine the best features of web and native apps. They provide a native-like experience to users, including the ability to work offline, send push notifications, and access device capabilities, all while being accessible through a web browser.
What is the role of Service Workers in PWAs?
Service Workers are a powerful JavaScript API that enable various functionalities for PWAs. They perform tasks in the background, such as offline caching, background synchronization, and push notifications. Service Workers greatly enhance the performance, reliability, and user experience of PWAs.
How do I get started with building PWAs?
To get started with building PWAs, you need to understand the fundamental requirements. This includes creating a web app manifest, registering a service worker, and considering responsive design. You can follow step-by-step instructions to set up a basic PWA environment and explore best practices for PWA development.
How can Service Workers enhance my PWAs?
Service Workers can enhance your PWAs by enabling advanced features such as offline support, background synchronization, and push notifications. They allow your PWA to function even when the user is offline, sync data in the background, and send notifications to keep users engaged.
What are the strategies for optimizing performance in PWAs?
To optimize performance in PWAs, you can employ various strategies. These include improving loading speed, reducing network usage, and optimizing rendering. Service Workers can help by caching critical resources and ensuring a smooth and responsive user experience.
What security considerations should I keep in mind for PWAs?
Security is crucial for PWAs. It’s important to mitigate potential security risks and protect sensitive user information. Best practices include securing Service Workers, handling offline data securely, and implementing proper authentication and authorization mechanisms.
How do I test and debug my PWAs?
Testing and debugging are essential aspects of PWA development. There are tools and techniques available for testing PWAs across multiple devices and browsers. You can also debug Service Workers and troubleshoot common issues that may arise during development.
How do I deploy and publish my PWAs?
Deploying and publishing PWAs involves packaging them for different platforms and distributing them through various channels. You can optimize your PWA for app store listings and ensure a seamless installation experience for users. The deployment process may vary depending on the platform and distribution channels you choose.
What are the advancements and future prospects of PWAs and Service Workers?
PWAs and Service Workers are constantly evolving. It’s important to stay up-to-date with the latest standards, APIs, and browser support. Explore emerging technologies and trends that are shaping the landscape of PWAs and be prepared to adapt to changing user expectations. Happy coding!
Next tutorial: Mastering GraphQL and Apollo Client in Javascript: A Comprehensive Guide
1 Comment