Routing in React is a fundamental concept that enables navigation between different components or pages within a single-page application. It facilitates the creation of dynamic and interactive web applications by providing the user with a seamless navigation experience.
In this complete guide, we will cover all the essential aspects of routing in React. By the end of this article, you will have a comprehensive understanding of how to implement routing in your React applications, including setting up routing, navigating between routes, handling dynamic routes, implementing nested routes, and optimizing routing performance.
Whether you’re a beginner or an experienced developer, this guide is designed to provide you with all the necessary information and insights to master routing in React. So let’s dive into this complete guide to routing in React!
What is Routing?
Routing is a fundamental concept in web development that involves directing network traffic between different endpoints. In the context of React, routing enables navigation between different components or pages within a single-page application.
Routing is essential to creating dynamic and interactive web applications that provide a seamless user experience.
When a user clicks on a link or enters a URL in the address bar, the browser sends a request to the server for the corresponding webpage. The server then responds with the HTML, CSS, and JavaScript files needed to render the page in the browser.
With routing, you can specify which components should be rendered based on the requested URL. This enables you to create complex user interfaces with multiple pages or views that can be loaded dynamically without a full-page refresh.
Setting Up Routing in React
Implementing routing in your React application does not have to be a daunting task. With the right approach and the necessary tools, you can quickly set up routing and start building dynamic and interactive applications. In this section, we will guide you through the process of setting up routing in your React application using the React Router library.
Installing React Router
The first step in setting up routing in your React application is to install the React Router library. You can do this by running the following command in your terminal:
npm install react-router-dom
This will install the necessary packages and dependencies for React Router to work in your application.
Configuring Routes
Once you have installed the React Router library, you can start configuring your routes. In your main App.js file, you will need to import the necessary components and define your routes using the Route component from React Router. Here is an example of how this might look:
Path | Component |
---|---|
/ | HomePage |
/about | AboutPage |
/contact | ContactPage |
In this example, we have defined three routes: the home page, the about page, and the contact page. The Path defines the URL path, and the Component defines the corresponding component that will be displayed when the user navigates to that path. You can define as many routes as you need for your application.
Integrating Routing with Components
Now that you have defined your routes, you need to integrate routing with your components. To do this, you can use the Link component from React Router. Here is an example of how this might look:
- Import Link from ‘react-router-dom’
- Wrap your component with a Switch component. This ensures that only one component is rendered at a time.
- Use the Link component to create clickable links that navigate to your defined routes.
Here is an example:
<Link to="/about">About</Link>
This creates a clickable link that navigates to the about page when clicked.
With these steps, you have successfully set up routing in your React application. In the next section, we will explore different methods and techniques for navigating between routes.
Navigating Between Routes
Once you have set up routing in your React application, the next step is to understand how to navigate between routes. There are different techniques for achieving this, and we will explore the most common ones in this section.
Using Links
The simplest way to navigate between routes is by using links. You can use the Link component provided by React Router to create links within your components. Here is an example:
import { Link } from 'react-router-dom';
function Home() {
return (
<div>
<h2>Home</h2>
<ul>
<li><Link to="/about">About</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</div>
);
}
In this example, we have used the Link component to create two links: one to the About component and one to the Contact component. By clicking on these links, the user will be taken to the corresponding routes.
Programmatic Navigation
In some cases, you may want to navigate programmatically, for example, after a form submission or some other user action. To achieve this, you can use the history object provided by React Router. Here is an example:
import { useHistory } from 'react-router-dom';
function Login() {
const history = useHistory();
function handleLogin() {
// Perform login logic
history.push('/dashboard');
}
return (
<div>
<h2>Login</h2>
<button onClick={handleLogin}>Login</button>
</div>
);
}
In this example, we have used the useHistory hook to access the history object. We have then defined a function handleLogin that performs the login logic and navigates to the Dashboard component by calling history.push.
Handling Dynamic Routes
When dealing with dynamic routes, such as routes that contain parameters, you need to handle them differently. React Router provides a way to extract parameters from the route URL using the useParams hook. Here is an example:
import { useParams } from 'react-router-dom';
function UserProfile() {
const { username } = useParams();
return (
<div>
<h2>{username}</h2>
<p>This is the profile page for {username}</p>
</div>
);
}
In this example, we have used the useParams hook to extract the username parameter from the route URL. We have then used it to display the profile page for that user.
Summary
By using links, programmatic navigation, and handling dynamic routes, you can navigate between different routes in your React application. These techniques are essential for creating a seamless user experience and providing easy access to different parts of your application.
Route Parameters and Dynamic Routing
Route parameters are a powerful feature in React routing that allows you to create dynamic routes and pass data to your components. With route parameters, you can make your application more flexible and intuitive, providing users with a personalized experience.
What are Route Parameters?
Route parameters are placeholders in the URL that can be used to pass data to a specific component. When a user navigates to a route with parameters, React extracts the data from the URL and provides it to the corresponding component. This allows you to create dynamic routes that can adapt to different scenarios.
How to Use Route Parameters?
Route parameters are defined by adding a colon followed by an identifier to the path of a specific route. For example, if you want to create a route that displays a specific user’s profile, you can define the path as /users/:id. The :id part is the parameter, and it can be accessed from the component using the props.match.params object.
Here’s an example of how to define a route with parameters:
<Route path="/users/:id" component={UserProfile} />
In this example, the UserProfile component will receive the id parameter via the props.match.params object. You can then use this parameter to fetch data from your backend or to render content based on the user’s ID.
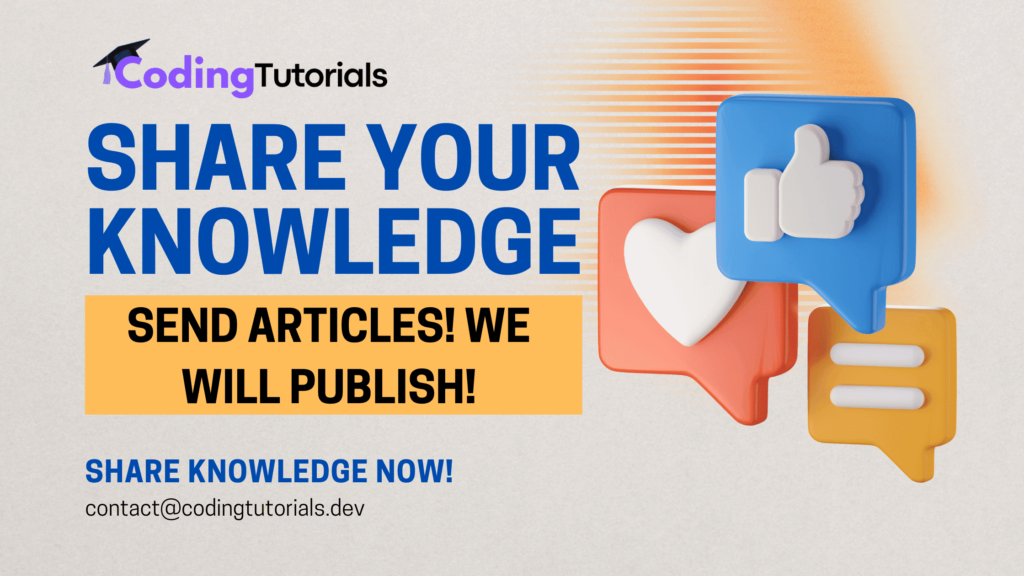
Nesting Parameters
You can also nest parameters within routes to create more complex routing scenarios. For example, if you have a route that displays a list of posts, you can create a nested route that displays a specific post using the post’s ID:
<Route path="/posts" component={PostList} />
<Route path="/posts/:id" component={Post} />
In this example, the PostList component will display a list of posts, and the Post component will display a specific post when the user navigates to /posts/:id. Again, the id parameter can be accessed via the props.match.params object.
Handling Invalid Parameters
When working with route parameters, it’s important to handle scenarios where the user enters invalid data or navigates to a non-existent page. One way to handle this is to use a conditional statement to check if the parameter exists, and display an appropriate message if it doesn’t:
function Post(props) {
const postId = props.match.params.id;
if (postId && postId > 0) {
// fetch data and render post
} else {
return <div>Invalid Post ID</div>;
}
}
In this example, the Post component checks if the id parameter exists and is valid (i.e., greater than 0). If the parameter is invalid, the component returns a message indicating that the ID is invalid.
Route parameters are a powerful tool for creating dynamic routes and passing data between components. By using route parameters in your React application, you can provide users with a more personalized and intuitive experience.
Nested Routes and Route Hierarchy
In this section, we will explore the implementation of nested routes and route hierarchy in React. Nested routes allow for a more organized and intuitive routing structure, enabling components to be nested within each other and creating a hierarchical relationship between routes. This can simplify navigation and provide a clearer overview of the application’s structure.
Creating Nested Routes
To create a nested route in React, you can simply define a sub-route within a parent route. The sub-route will inherit the path of the parent route, creating a hierarchical structure. Here’s an example:
<Route path="/parent">
<Route path="/child" component={ChildComponent} />
</Route>
In this example, the “/child” route is nested within the “/parent” route, creating a hierarchical relationship between the two.
Handling Nested Routes in Components
To handle nested routes within components, you can use the “props.children” property to render the nested components. Here’s an example:
const ParentComponent = (props) => {
return (
<div>
Parent Component
{props.children}
</div>
);
};
const ChildComponent = () => {
return <div>Child Component</div>;
};
In this example, the “ParentComponent” is passed the “props.children” property, which will render the “ChildComponent” when the “/child” route is accessed.
Benefits of Route Hierarchy
The use of nested routes and route hierarchy in React can provide several benefits for your application, including:
- Improved organization and structure of your application
- Easier navigation within your application
- More intuitive and user-friendly routing
- Reduced complexity and increased scalability
In conclusion, nested routes and route hierarchy can greatly enhance the routing structure of your React application. By creating a hierarchical relationship between routes, you can simplify navigation and provide a more organized and intuitive user experience.
Protected Routes and Authentication
If you’re building a web application that requires user authentication, protecting certain routes and content is crucial to ensuring the security and privacy of your users.
React provides developers with the ability to create protected routes that can only be accessed by authenticated users. Let’s take a look at how to implement this feature in your application.
Setting Up Protected Routes
The first step in setting up protected routes is to create a component that will act as a wrapper for the protected content. This component will check if the user is authenticated and authorized to access the content, and if not, redirect them to the login page.
Here’s an example of what a protected route component might look like:
<Route path="/protected" render={() => (
authenticated ? (
<ProtectedComponent />
) : (
<Redirect to="/login" />
)
)} />
In this example, the Route
component defines the path for the protected route and uses render()
to check if the user is authenticated using a boolean variable called authenticated
.
If the user is authenticated, the ProtectedComponent
is rendered, and if not, the user is redirected to the login page using the Redirect
component.
Implementing Authentication
Before you can use protected routes, you need to implement authentication in your application. There are many ways to implement authentication in React, but one common method is to use JSON Web Tokens (JWT).
When the user logs in, the server generates a unique JWT and sends it to the client. The client can then store the JWT in local storage or a cookie and include it in subsequent requests to the server.
Here’s an example of how to implement authentication using JWT:
- The user submits their login credentials to the server.
- The server verifies the credentials and generates a JWT.
- The server sends the JWT to the client.
- The client stores the JWT in local storage or a cookie.
- The client includes the JWT in the header of subsequent requests to the server.
- The server verifies the JWT and grants access to protected routes and content.
Route Guards and Authorization
Route guards are a powerful tool in React routing that allows you to restrict access to certain routes based on user roles or permissions. This means that unauthorized users will not be able to access protected routes, providing an additional layer of security to your application.
To implement route guards, you will need to integrate a middleware that handles authentication and authorization logic. This middleware will check whether the user is allowed to access the route and either allow or deny access accordingly, redirecting unauthorized users to a login page or an error page.
There are different strategies for implementing route guards and authorization, depending on your application’s requirements and complexity. Some common techniques include:
- Role-based access control: This technique involves assigning roles or permissions to users and defining which routes can be accessed by each role. For instance, an admin role may have access to all routes, while a guest role may only have access to the landing page and a few other public routes.
- Token-based authentication: This technique involves generating tokens for authenticated users and verifying these tokens on subsequent requests. Tokens can be stored in cookies or local storage and can be used to determine the user’s access level and permissions.
- IP-based access control: This technique involves whitelisting or blacklisting IP addresses based on their origin or other criteria. This can be useful to prevent access from certain regions or countries that may pose a security risk or to allow access only from trusted sources.
Implementing route guards and authorization requires careful planning and testing to ensure that your application is secure and user-friendly. Make sure to consider all possible scenarios and edge cases and to provide clear feedback to users when access is denied.
Optimizing Routing Performance
Routing is an essential part of any React application, and as your application grows, it’s important to ensure that routing remains efficient and responsive. In this section, we will cover some tips and techniques for optimizing routing performance in your React applications.
Code Splitting
One of the most effective ways to improve routing performance is by implementing code splitting. Code splitting involves breaking down your code into smaller chunks that can be loaded on demand, rather than loading the entire application at once. By splitting your code into smaller chunks, you can reduce the amount of time it takes to load your application, which can significantly improve routing performance.
Lazy Loading
Lazy loading is another technique that can be used to improve routing performance. Lazy loading involves dynamically loading components only when they are needed, rather than loading all components at once. This can help reduce the overall size of your application and improve routing performance by minimizing the amount of code that needs to be loaded.
Caching
Caching is a technique that can be used to store frequently accessed data in memory, so that it can be accessed more quickly in the future. By caching frequently accessed data, you can reduce the amount of time it takes to retrieve data from the server, which can significantly improve routing performance.
Minimizing DOM Updates
Another way to optimize routing performance is by minimizing the number of DOM updates that occur when navigating between routes. Each time a route is rendered, the entire DOM tree must be updated, which can be a time-consuming process. By minimizing the number of DOM updates that occur, you can improve routing performance and create a smoother navigation experience for your users.
Conclusion
Routing is an essential aspect of web development, and React provides a powerful set of tools and techniques for implementing routing in your applications. By following the comprehensive guide presented in this article, you can master the fundamentals of routing in React and unlock the full potential of this powerful library.
Summary of Guide
We began by introducing the concept of routing in React and providing an overview of its importance in web development. We then delved into the process of setting up routing in your React application, covering installation, configuration, and integration with your components.
Next, we explored different methods and techniques for navigating between routes in React, including the use of links, programmatic navigation, and dynamic routes. We also covered the use of route parameters to enable dynamic routing, as well as the implementation of nested routes and route hierarchy.
We then discussed the implementation of protected routes and authentication, including the use of middleware and user redirection. We also covered the use of route guards and authorization to restrict access to certain routes based on user roles or permissions.
Finally, we provided tips and techniques for optimizing the performance of routing in React, including code splitting, lazy loading, and caching.
Unlocking the Power of Routing in React
By mastering routing in React, you can create dynamic and interactive web applications with seamless navigation. Whether you are building a simple blog or a complex e-commerce platform, the techniques presented in this guide can help you achieve your goals.
So go ahead and start implementing routing in your React applications today. With the power of React and the guidance provided in this article, you can take your web development skills to the next level!
FAQ
What is routing in React?
Routing in React refers to the process of managing and navigating between different components or pages within a single-page application. It allows users to move between different views or sections of an application without having to reload the entire page.
Why is routing important in web development?
Routing is crucial in web development as it enables the creation of dynamic and interactive user interfaces. It allows for the seamless navigation between different parts of an application, enhancing the user experience and providing a smooth flow of information.
How do I set up routing in React?
To set up routing in React, you need to install the necessary packages, such as React Router, and configure the routes in your application. This involves defining the paths and corresponding components for each route in a centralized routing configuration file or within the components themselves.
How can I navigate between routes in React?
There are multiple ways to navigate between routes in React. You can use links, such as the “ component provided by React Router, to create clickable navigation elements. Additionally, you can perform programmatic navigation using history objects or hooks provided by React Router.
How can I create dynamic routes in React?
Creating dynamic routes in React involves using route parameters. These parameters are placeholders within the route path that can be accessed and used within the component associated with that route. By extracting and utilizing route parameters, you can make your routes more flexible and dynamic.
How do I handle nested routes in React?
Nested routes in React allow for a hierarchical routing structure, where child routes are nested within parent routes. To handle nested routes, you need to define the parent-child relationships in your routing configuration and ensure that the appropriate components are rendered based on the current route.
How can I protect certain routes and implement authentication in React?
To protect routes and implement authentication in React, you can use techniques such as route guards and authentication middleware. These mechanisms allow you to restrict access to certain routes based on user authentication status or permissions. Unauthorized users can be redirected to a login page or denied access altogether.
How can I optimize the performance of routing in React?
To optimize routing performance in React, you can employ strategies such as code splitting, lazy loading, and caching. Code splitting involves splitting your application’s code into smaller chunks, allowing for faster initial loading times. Lazy loading defers the loading of components until they are actually needed. Caching can help reduce unnecessary requests and improve the overall speed of your application. Happy coding!
Next tutorial: How to Start Coding: An Ultimate Guide to beginners
Leave a Reply