Welcome to our comprehensive guide on server side rendering in React! In this tutorial, we’ll explore everything you need to know about implementing server side rendering in your React projects. We’ll cover the benefits of this powerful technique, including improved performance and SEO friendliness, and delve into the various strategies to optimize your application’s performance using SSR.
Whether you’re a seasoned React developer or just starting out, this guide is for you. We’ll start by explaining the fundamental concepts of server side rendering and how it differs from client side rendering. Then, we’ll walk you through the step-by-step process of implementing SSR, covering everything from handling data and state, troubleshooting issues, and even tackling advanced techniques like authenticated routes and server-side caching. By the end of this guide, you’ll be proficient in server side rendering in React and ready to take your applications to the next level.
So, if you’re ready to improve your React app’s performance, create SEO-friendly websites, and master the art of server side rendering, let’s dive in and get started!
Understanding Server Side Rendering in React
Before diving into the implementation details, it’s important to have a clear understanding of what server side rendering (SSR) is in the context of React.
What is Server Side Rendering in React?
Server Side Rendering (SSR) is the technique of rendering your React application on the server, rather than in the client browser. When a user requests a page from your React app, the server sends back a fully-rendered HTML page, rather than an empty document with JavaScript that must be executed to display content, as in client-side rendering.
How Does Server Side Rendering Differ from Client Side Rendering?
Client side rendering involves rendering your React app in the user’s browser using JavaScript. This means the browser must download the JavaScript bundle, render the HTML page, and then execute the JavaScript to render the content. This process can be slower and less SEO-friendly compared to SSR.
Why Use Server Side Rendering in React?
React SSR offers several benefits, including:
- Better SEO performance: Search engines can crawl and index your pages more easily, as they receive fully rendered HTML pages. This improves your website’s visibility in search results.
- Faster initial load times: Since the server sends back a fully rendered page, the user sees content faster, improving user experience.
- Enhanced performance on low-end devices: SSR ensures the user’s device only has to execute the minimal amount of JavaScript needed, which can be especially helpful on less powerful devices.
The Benefits of Server Side Rendering in React
Server side rendering in React offers several key advantages compared to client-side rendering. Let’s take a look at some of the most significant benefits:
Improved Initial Load Times
One of the most significant advantages of server side rendering is improved initial page load times. By pre-rendering content on the server before sending it to the client, we can significantly reduce the time it takes for the user to see our app’s content.
With client-side rendering, the browser needs to download all the JavaScript files before it can render the page. This can lead to a delay in initial load times, especially for larger applications. With server side rendering, this delay is significantly reduced, resulting in a more seamless user experience.
Better SEO
Server side rendering also offers significant benefits for search engine optimization (SEO). By pre-rendering content on the server, search engine crawlers can easily index our pages, resulting in better search engine visibility.
Additionally, pre-rendered pages are more likely to be shared on social media platforms, as they provide a more complete and visually engaging experience for the user.
Enhanced User Experience
Server side rendering enables a more seamless user experience, especially on mobile devices and for users with slower internet connections. By reducing the amount of JavaScript that needs to be downloaded and executed on the client, we can improve the overall performance of our app and provide a more responsive user interface.
Furthermore, server side rendering allows us to deliver content to users even if they have JavaScript disabled in their browser, ensuring that our app is accessible to as many users as possible.
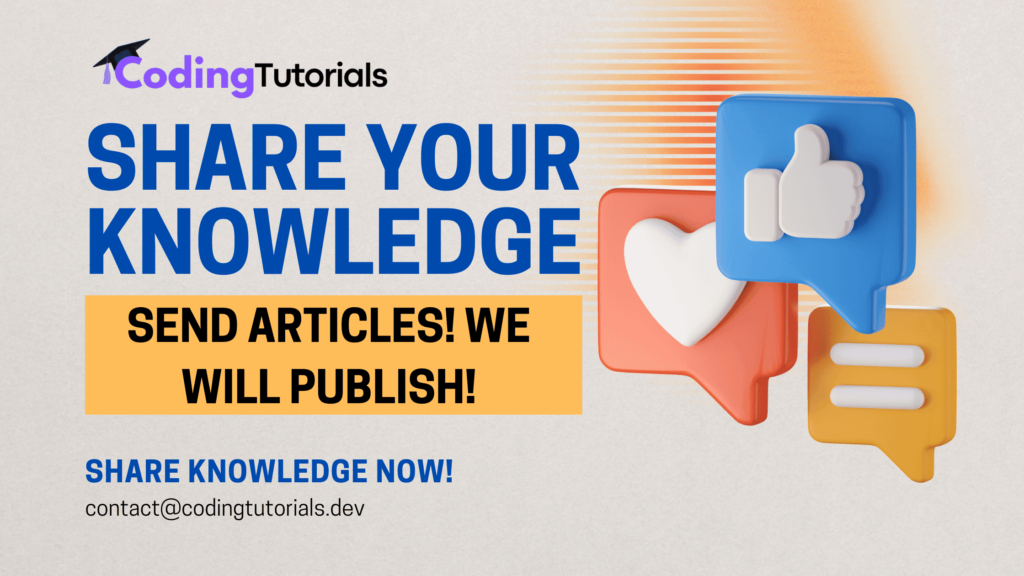
Getting Started with Server Side Rendering in React
Implementing server side rendering (SSR) in React projects can be intimidating for developers who are new to the technique. However, with the right guidance, the process can be straightforward and rewarding. In this section, we will provide a step-by-step tutorial for implementing SSR in a React project.
Step 1: Set Up Your Server
The first step to implementing SSR is to set up a server that can render your React components. There are several server-side rendering frameworks that you can use, including Next.js, Razzle, and After.js. For the purpose of this tutorial, we will be using Next.js, a popular framework that makes SSR easy and intuitive.
To get started with Next.js, install it using npm:
npm install next react react-dom
Once you’ve installed Next.js, create a new Next.js app by running the following command:
npx create-next-app
This will generate a new Next.js app with the necessary files and folders.
Step 2: Create a Basic React Component
The next step is to create a basic React component that we can use for SSR. In this example, we’ll create a simple “Hello World” component:
function HelloWorld() {
return (
<div>Hello World!</div>
);
}
Save this component in a new file called “HelloWorld.js” in the “components” folder of your Next.js app.
Step 3: Create a Server-Side Rendered Page
The final step is to create a server-side rendered page that uses the “HelloWorld” component. In Next.js, you can create server-side rendered pages by creating a file in the “pages” directory. Create a new file called “index.js” in the “pages” directory and add the following code:
import HelloWorld from '../components/HelloWorld';
export default function Home() {
return (
<div>
<HelloWorld />
</div>
);
}
Step 4: Start the Server
With your “Hello World” component and server-side rendered page in place, you’re ready to start the server and test your app. To start the server, run the following command:
npm run dev
This will start the Next.js development server, which you can access at http://localhost:3000. If all goes well, you should see your “Hello World” component rendered on the page.
Congratulations! You have successfully implemented server side rendering in your React project using Next.js. This is just the beginning, and there are many more features and optimizations you can explore with SSR. Stay tuned for our next section on performance optimization with server side rendering in React.
Performance Optimization with Server Side Rendering in React
Server side rendering (SSR) offers a significant improvement in performance for React applications. By rendering the initial HTML on the server, the browser can start rendering the page content faster, resulting in a shorter time-to-first-paint and better perceived performance.
However, simply implementing SSR does not guarantee optimal performance. In this section, we will explore various techniques and strategies to optimize performance with SSR, ensuring that your React application is lightning fast.
Code Splitting
Code splitting is a technique that breaks down your application code into smaller chunks, reducing the initial load time for users. With SSR, you can use code splitting to ensure that only the necessary code is loaded on the server, improving the time-to-first-paint and perceived performance.
React provides several code-splitting techniques, including dynamic and static imports, which you can use to further optimize performance. Dynamic imports allow you to request only the necessary code on demand, while static imports enable you to load code during the initial SSR render.
Server-side Caching
Caching is an essential technique for improving performance in SSR. By caching frequently accessed data on the server, you can reduce the number of requests made by the client, improving performance and reducing server load.
Pros | Cons |
---|---|
Reduces server load and client requests. | May result in stale data if cache is not refreshed. |
Improves performance and perceived performance. | Requires management of cache invalidation and updates. |
There are several server-side caching strategies you can use, including memory caching, file caching, and database caching, each with its own pros and cons. By combining caching with code splitting, you can achieve even better performance, reducing load times and improving user experience.
Minimizing Network Requests
Minimizing network requests is another essential technique for improving performance in SSR. By reducing the number of requests made by the client and server, you can shorten the load time and reduce user frustration.
One effective way to minimize network requests is to bundle all necessary assets, such as CSS and JavaScript files, into a single request. By sending all files in a single response, you can avoid the overhead of multiple requests and reduce the load time.
Caching on the Client
Finally, you can also improve performance by caching data on the client. By storing frequently accessed data in the client’s cache, you can reduce the number of requests made to the server, improving load times and reducing server load.
React provides several mechanisms for client-side caching, including React’s built-in useContext and useReducer hooks, as well as third-party libraries like Redux. By combining client-side caching with server-side caching and code splitting, you can achieve optimal performance and provide a seamless user experience.
Handling Data and State in Server Side Rendering
When it comes to server side rendering in React, managing data and state can be challenging. However, with the right techniques and best practices, you can optimize your application’s performance and user experience.
Fetching Data on the Server
One of the primary benefits of server side rendering is the ability to fetch data on the server and pre-populate components with data before sending them to the client. This can significantly reduce the time it takes for your application to render and enhance user experience. To achieve this, you can use libraries or built-in APIs to fetch data during server-side rendering.
Synchronizing Data with the Client
After data has been fetched on the server, it is essential to synchronize it with the client to ensure a seamless user experience. You can achieve this by serializing the data and sending it to the client in the initial HTML response, making it available to React components as they are hydrated on the client. This approach ensures that data is always up-to-date and eliminates the need for additional data fetching on the client.
Managing State
Server side rendering in React can also introduce state management challenges. Since components are rendered on both the server and client, you need to ensure that state is consistent between the two environments. One way to achieve this is by using a state management library or Redux. These tools allow you to manage state globally and ensure consistency across the entire application.
SEO-Friendly React Websites with Server Side Rendering
If you want your React website to rank well on search engines and attract more visitors, server side rendering (SSR) is a must-have. Implementing SSR in your React app will give you several benefits, including:
- Improved initial page load times: With SSR, your site’s HTML is generated on the server, which means that users will see the content more quickly than they would with client-side rendering.
- Better indexing: Search engines can crawl and index your content more easily with SSR, which can lead to higher rankings in search results.
- Enhanced social media sharing: When users share links from your site on social media, the content will be fully rendered and available, making it more attractive to potential visitors.
To get started with implementing SSR in your React app, you can follow our detailed React server rendering tutorial in section 4. Once you have implemented SSR, you can enjoy the benefits of a faster, more responsive, and more attractive website that attracts more visitors and offers a better user experience.
Handling Dynamic Routes in Server Side Rendering
When it comes to server side rendering in React, handling dynamic routes can be a challenge. This is because, unlike static routes, dynamic routes require rendering of data on the server, which can be a complex process. However, with the right techniques, you can efficiently handle dynamic routes in SSR.
Parameterized URLs
Parameterized URLs are a common feature in React applications, especially those that require user interaction. With parameterized URLs, the URL contains query parameters that define the content to be displayed.
To handle parameterized URLs in SSR, you need to identify the parameters in the URL and fetch their corresponding data on the server. This data can then be passed to the React component for rendering.
Route-Based Data Fetching
Another common technique for handling dynamic routes in SSR is route-based data fetching. With this approach, each route has its own set of data that needs to be fetched and rendered on the server.
To implement route-based data fetching, you need to create a server-side route map that defines the data requirements for each route. This map can then be used to fetch the required data on the server and pass it to the corresponding component for rendering.
Technique | Advantages |
---|---|
Parameterized URLs | – Easy to set up – Ideal for simple applications – Allows for passing of data through the URL |
Route-Based Data Fetching | – Allows for fine-grained control over data fetching – Ideal for complex applications – Enables efficient data management on the server |
Overall, handling dynamic routes in SSR requires careful planning and attention to detail. By choosing the right techniques and implementing them correctly, you can ensure that your application is optimized for performance and user experience.
Troubleshooting and Debugging Server Side Rendering Issues
While server side rendering is an incredibly powerful technique, it can sometimes present challenges and issues. In this section, we’ll cover some common problems you might encounter with React SSR and how to troubleshoot them.
Identifying Performance Bottlenecks
One of the most significant benefits of server side rendering is improved performance. However, it’s possible to encounter bottlenecks that slow down your application. When you’re dealing with a slower than expected response time, you need to start by identifying the root cause of the problem.
Google’s Lighthouse tool is an excellent resource to help identify performance bottlenecks. However, other tools like Chrome DevTools and WebPageTest.org are also valuable for highlighting potential optimization opportunities.
Fixing Rendering Issues
When it comes to rendering issues, there are a few strategies that can help. One approach is to use React’s built-in debugging tools to inspect your application’s state during the rendering process. You can also use the Google Chrome DevTools to identify whether there are any issues with your CSS or JavaScript code.
If you’re experiencing issues with server side rendering, it’s important to make sure that your application is rendering correctly on the client side. Check that your React components are rendering as expected and that there are no issues with state management.
Testing and Refining SSR
As with any technique, testing and refinement are a critical part of making SSR work for your application. It’s important to test your application across multiple browsers and devices to ensure that it’s responsive and works as expected. You can also use tools like Google Analytics to measure the impact of server side rendering on your performance metrics.
Refining your implementation can help you get the most out of server side rendering. Experiment with different caching strategies, code splitting techniques, and data fetching mechanisms to optimize performance and deliver the best possible user experience.
Wrapping Up
By following these best practices, you’ll be well-equipped to troubleshoot and debug server side rendering issues in your React applications. Remember to identify performance bottlenecks, fix rendering issues, test and refine your implementation, and don’t hesitate to seek help from the React community if you’re stuck.
Next up, we’ll explore some advanced server side rendering techniques you can use to take your application to the next level.
Advanced Server Side Rendering Techniques
Now that you have mastered the basics of server side rendering in React, it’s time to take your skills to the next level with advanced techniques and optimizations. In this section, we will explore some of the most powerful and versatile tools for implementing server side rendering in your React applications. Follow along with our detailed react ssr implementation tutorial to gain hands-on experience.
Server-Side Caching for Improved Performance
Server-side caching can significantly improve the performance of your React application by reducing the number of requests made to the server. In this technique, the server stores a cached version of the response for a particular request and serves that to subsequent requests instead of generating a new response every time. Caching can be implemented with various strategies, such as memory caching, file caching, and CDN caching. We will explore these strategies in detail in our react server rendering tutorial.
Handling Authenticated Routes on the Server
Handling authenticated routes on the server can be challenging, as it requires the server to authenticate the user before rendering the page. One way to do this is by passing the authentication token as a prop to the server-rendered components. Another technique is to use a state management library like Redux or MobX to manage the authentication state. We will demonstrate these techniques in our react ssr implementation tutorial.
Optimizing Code Splitting for Faster Load Times
Code splitting is a powerful technique for reducing the initial load time of your React application by splitting the code into smaller chunks that can be loaded on demand. However, implementing code splitting on the server side can be tricky. In this section, we will explore how to optimize code splitting for server side rendering to ensure lightning-fast load times.
Conclusion
That concludes our comprehensive guide on mastering server side rendering in React. By now, you should have a solid understanding of what server side rendering is, its benefits, and how to implement it in your React projects.
Takeaways
Firstly, we explored how server side rendering improves initial page load times, enhances search engine optimization, and enables a more seamless user experience. Then, we dove into the step-by-step process of implementing SSR in a React project and optimizing performance with various techniques and strategies.
We also covered best practices for handling data and state, creating SEO-friendly React websites, and handling dynamic routes. And lastly, we discussed common troubleshooting and debugging techniques for SSR in React and advanced optimizations.
Start Your Practice
We hope you found this React server rendering tutorial helpful and feel confident in using server side rendering in your future projects. Remember, practice makes perfect, so keep experimenting and building your skills in server side rendering with React.
Thank you for reading and happy server side rendering!
FAQ
What is server side rendering in React?
Server side rendering (SSR) in React is the process of rendering React components on the server and sending the fully rendered HTML to the client. This allows search engines to crawl and index the content, improves initial page load times, and enhances SEO for React websites.
What are the benefits of server side rendering in React?
Server side rendering offers several benefits for React applications. It improves initial page load times, enhances search engine optimization by making content crawlable, and enables a more seamless user experience by delivering fully rendered HTML to the client.
How can I get started with server side rendering in React?
To get started with server side rendering in React, you can follow our step-by-step tutorial. It will walk you through the process of implementing SSR in a React project, helping you gain hands-on experience.
How can I optimize performance with server side rendering in React?
There are various techniques and strategies you can apply to optimize performance with server side rendering in React. From code splitting to caching, you can make your React application lightning fast. Learn more about these techniques in our performance optimization section.
How do I handle data and state in server side rendering?
Dealing with data and state is a crucial aspect of server side rendering in React. Our guide covers best practices for managing data and state in SSR, such as fetching data on the server and synchronizing it with the client. Explore this section to learn more.
How does server side rendering contribute to creating SEO-friendly React websites?
Server side rendering plays a vital role in creating SEO-friendly React websites. It improves search engine visibility, allows for better indexing, and enhances social media sharing. Discover how to make your React app shine in search engine rankings in our SEO-Friendly React Websites section.
How do I handle dynamic routes in server side rendering?
React applications often have dynamic routes that need to be rendered on the server side. Our guide explores techniques for handling dynamic routes in SSR, including parameterized URLs and route-based data fetching. Gain in-depth knowledge on this topic by diving into our section on dynamic routes.
What troubleshooting and debugging techniques are available for server side rendering in React?
Server side rendering can sometimes face challenges that require troubleshooting and debugging. Our guide covers common techniques for identifying performance bottlenecks and fixing rendering issues in SSR. Learn how to overcome hurdles and ensure a smooth SSR experience.
Are there any advanced server side rendering techniques I can apply in React?
Once you have a solid understanding of the basics, you can explore advanced techniques and optimizations for server side rendering in React. From server-side caching to handling authenticated routes, our guide covers advanced scenarios to help you level up your SSR skills.
What is the conclusion of this comprehensive guide on mastering server side rendering in React?
Congratulations! You have reached the end of our comprehensive guide on mastering server side rendering in React. We have covered everything from the basics to advanced techniques, equipping you with the knowledge to leverage SSR in your React projects. Now, go forth and create high-performing, SEO-friendly React applications! Happy coding!
Next tutorial: Firebase Integration for React Js : Real-Time Applications
Leave a Reply